Part 8 Testing React Applications – Testing styling
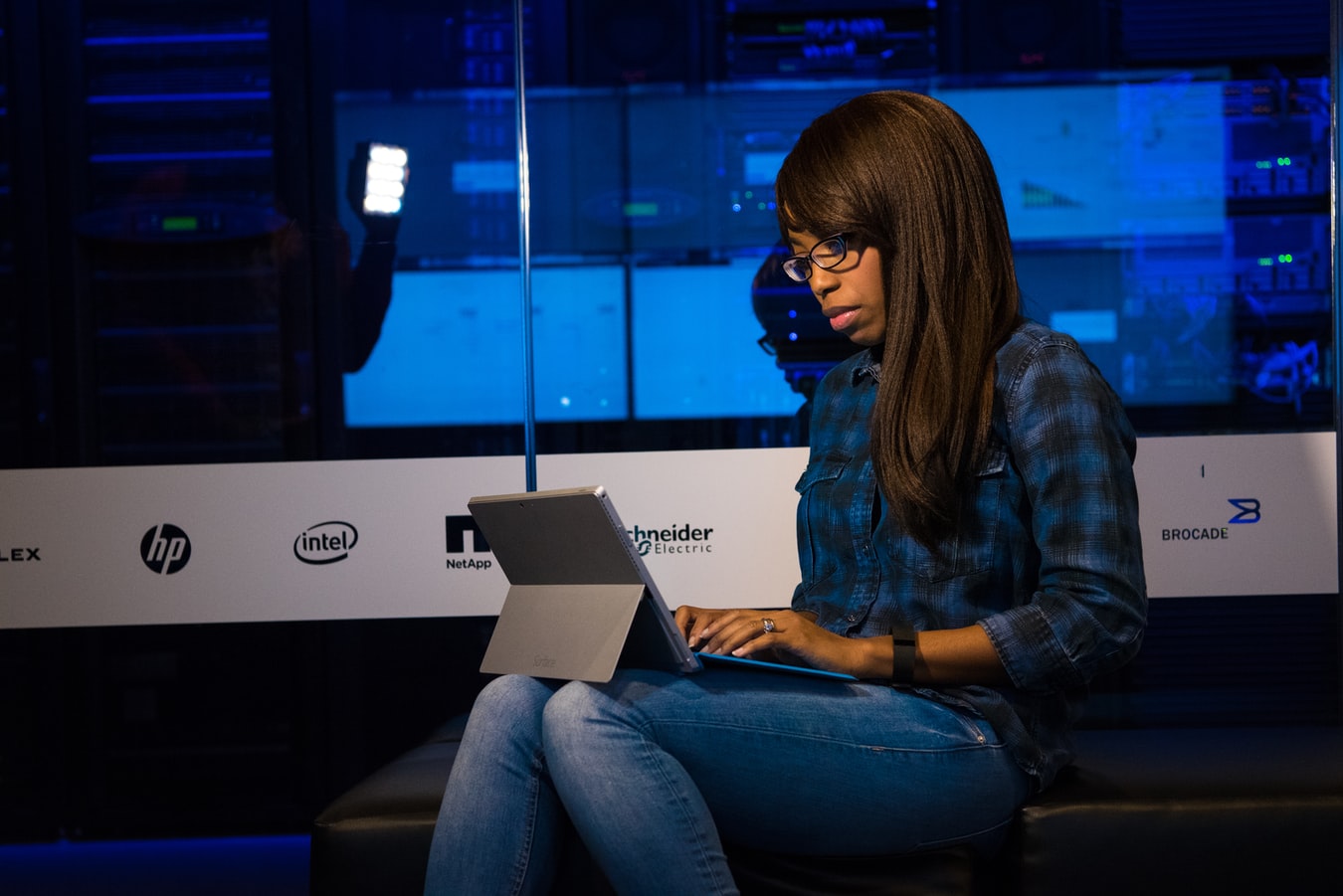
Hi, in our React component called Todo ( <Todo /> ) we have a prop called completed and if you see the Css style is related to that prop.
The “completed” prop handle the behavior of this component, so, if the user click this component in the browser then the completed prop will be true and the style class will be applied ‘line-through’ to this component.
Like this:
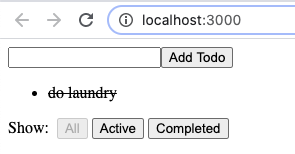
So this is our todo component code:
import React from 'react'
import PropTypes from 'prop-types'
const Todo = ({ onClick, completed, text }) => ( // loading1, loading2
<li
onClick={onClick}
style={{
textDecoration: completed ? 'line-through' : 'none'
}}
>
{text}
</li>
)
Todo.propTypes = {
onClick: PropTypes.func.isRequired,
completed: PropTypes.bool.isRequired,
text: PropTypes.string.isRequired
}
export default Todo
So next, is go to our test file called todo.test.js, so let’s create a new test suite for test styling code:
// Dependencies
import React from 'react'
// Components
import Todo from './Todo';
// Enzyme Dependencies
import Enzyme, { configure, shallow, mount } from 'enzyme';
import Adapter from 'enzyme-adapter-react-16';
// Creating adapter with Enzyme
Enzyme.configure({ adapter: new Adapter() });
// Test suite
describe("Styling behavior", () => {
// Simulating a function
const mockFn = jest.fn();
// Initializing the props of the component
const initialProps = {
onClick: mockFn,
completed: false,
text: "do laundry"
}
// The component
let component;
beforeEach(() => {
component = shallow(<Todo {...initialProps} />);
});
// First test case (unit test)
it("should not have linethrough style when Todo is incomplete", () => {
// Assertions
// Testing the component when completed is false (incomplete)
expect(component.props().style).toEqual({ textDecoration: 'none' });
});
// Second test case (unit test)
it("should have linethrough style when Todo is complete", () => {
component.setProps({ completed: true });
// Assertions
// Testing the component when completed is true (complete)
expect(component.props().style).toEqual({ textDecoration: 'line-through' });
});
});
Result:
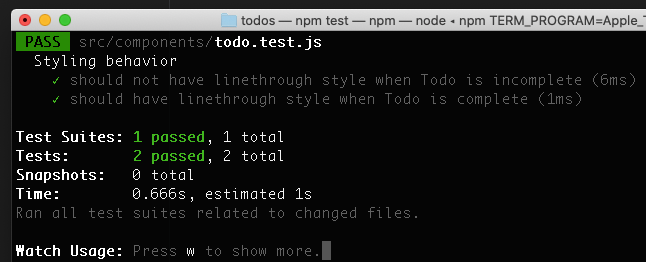
By Cristina Rojas.