Part 7 Testing React Applications – Refactor duplicate code
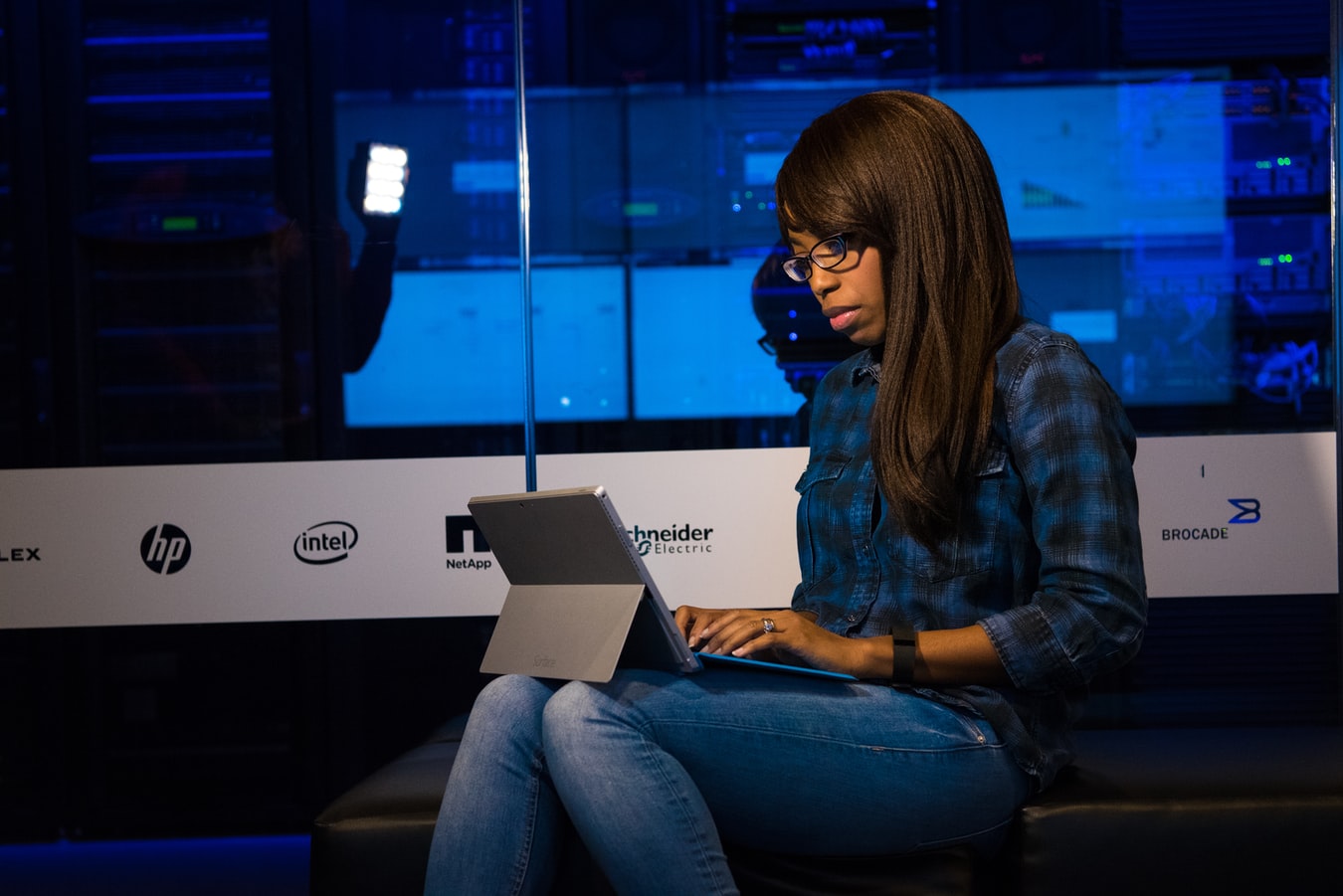
Hi, when we have duplicated code in our test cases we can refactor the duplicated code using some existing methods with Jest.
So in our test suite we have multiple test cases that have this line as repeated line
const component = shallow(<Todo {...initialProps} />)
So we can use the beforeEach and afterEach functions of Jest, so we can declare our repeated line of code inside the beforeEach this means that all the lines inside the beforeEach will be executed first and then all the test cases.
// 1 Individual Test case
it("should render 1 <Todo /> component", () => {
// shallow() method is from Enzyme
const component = shallow(<Todo {...initialProps} />);
// Assertions...
});
// 2 Individual Test Case
it("should render props correctly", () => {
// Using shallow from enzyme and passing all the props to the component
const component = shallow(<Todo {...initialProps} />)
// Assertions....
});
// 3 Individual Test case
it("should set props correctly", () => {
const component = shallow(<Todo {...initialProps} />);
So we can delete all the const component …line and insert this line into the beforeEach like this:
// Repeated code
let component;
beforeEach(() => {
component = shallow(<Todo {...initialProps} />);
});
Nice, now I’m going to copy all the code with the refactor
// Dependencies
import React from 'react'
// Components
import Todo from './Todo';
// Enzyme Dependencies
import Enzyme, { configure, shallow, mount } from 'enzyme';
import Adapter from 'enzyme-adapter-react-16';
// Creating adapter with Enzyme
Enzyme.configure({ adapter: new Adapter() });
/** Test suites **/
// First (1) test suite
describe("<Todo /> component Unit Tests", () => {
// jest.fn() is from Jest to simulate an onClick
const mockFn = jest.fn();
// Initializing the props of the component
const initialProps = {
onClick: mockFn,
completed: false,
text: "do laundry"
}
// Repeated code
let component;
beforeEach(() => {
component = shallow(<Todo {...initialProps} />);
});
// 1 Individual Test case
it("should render 1 <Todo /> component", () => {
// Assertions
expect(component).toHaveLength(1); // component is from Enzyme, expect(), toHaveLength() and toEqual() are from Jest
expect(component.find("li")).toHaveLength(1); // component.find() is from Enzyme
expect(component.props().children).toEqual("do laundry"); // component.props().children is from Enzyme
});
// 2 Individual Test Case
it("should render props correctly", () => {
// To know the component props
console.log(component.props());
// Assertions
expect(component.props().children).toEqual("do laundry");
});
// 3 Individual Test case
it("should set props correctly", () => {
// Changing the prop value
component.setProps({ text: "hola" });
// Assertions
expect(component.props().children).toEqual("hola");
});
// 4 Individual Test case
it("should call onClick handler when todo component is clicked", () => {
// Simulating onClick method
component.simulate("click"); // First click on the component
component.simulate("click"); // second click on the component
// Assertions
expect(mockFn).toHaveBeenCalledTimes(2); // expect(), mockFn and toHaveBeenCalledTimes are from Jest
});
});
Result:
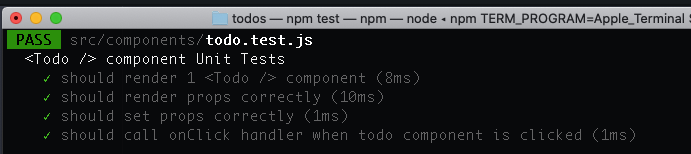
By Cristina Rojas.
Other posts: