Javascript Classes in ES6
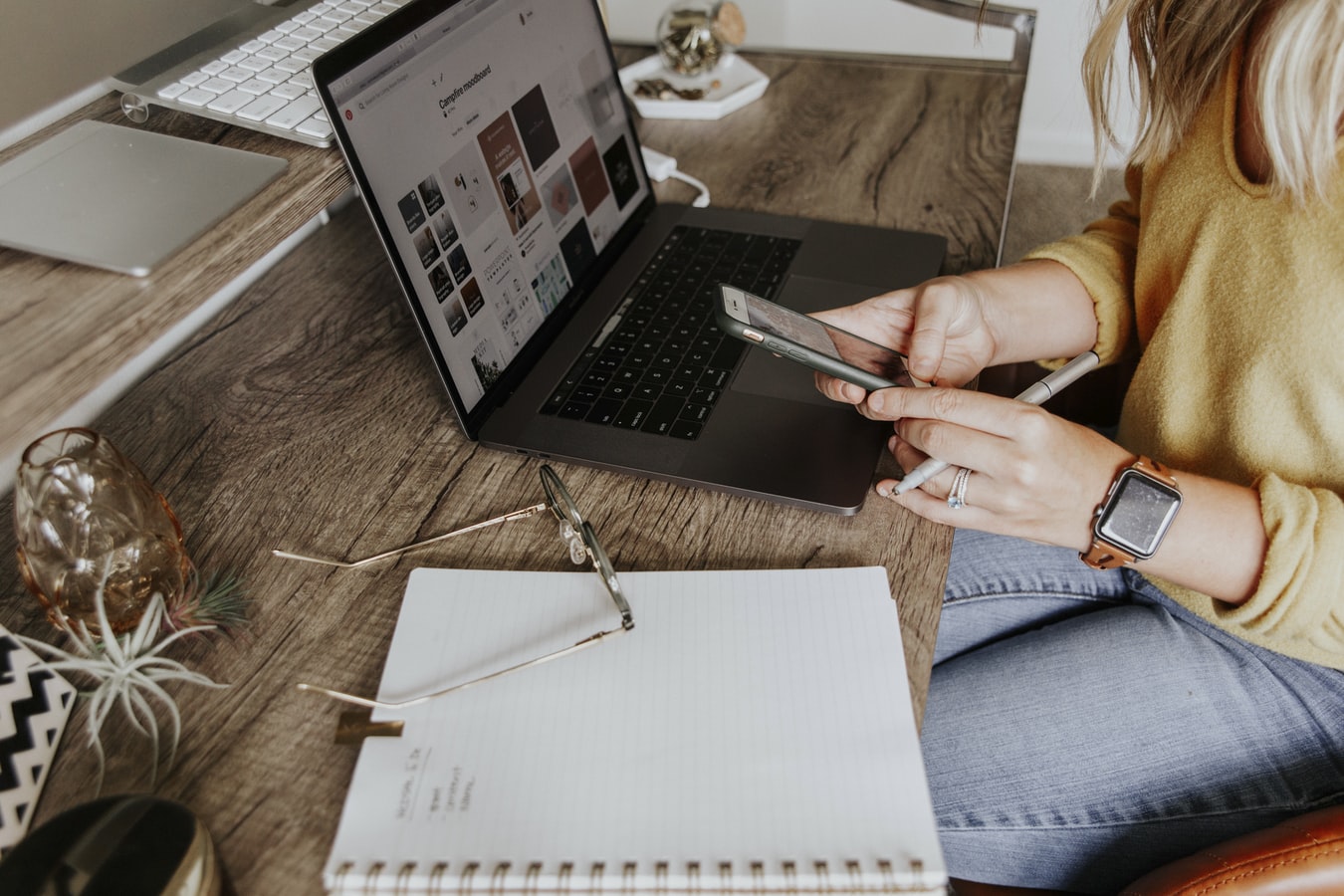
Hi, in ES6 version there is another way to create classes and is easy way to write classes.
The first thing that we need to do is use the “class” keyword to create the class, then declare the constructor and the methods that you will need like this example:
// ES6 class
class Book {
// declaring a constructor
constructor(title, author, year) {
this.title = title;
this.author = author;
this.year = year;
}
// Functions of our class
getBookSummary() { // to get all the book information
return `${this.title} was written by ${this.user} in year ${this.year}`;
}
getAge() { // to get the
const years = new Date().getFullYear() - this.year;
return `${this.title} is ${years} years old`;
}
revise(newYear) {
// Assinging new year to the book
this.year = newYear;
// and Assinging revised too
return this.revised = true;
}
// static method
static topBookStore() {
return 'Barnes & Noble';
}
}
// Initiatite a new object
const book1 = new Book('Book 1', 'Cristina Rojas', '2020');
// Using the revise method directly
console.log(book1);
book1.getAge();
book1.revise('2018')
console.log(book1);
// To call the static topBookStore, call the class then the method.
console.log(Book.topBookStore());
Result:

By Cristina Rojas.