HackerRank Sock Merchant Solution Js
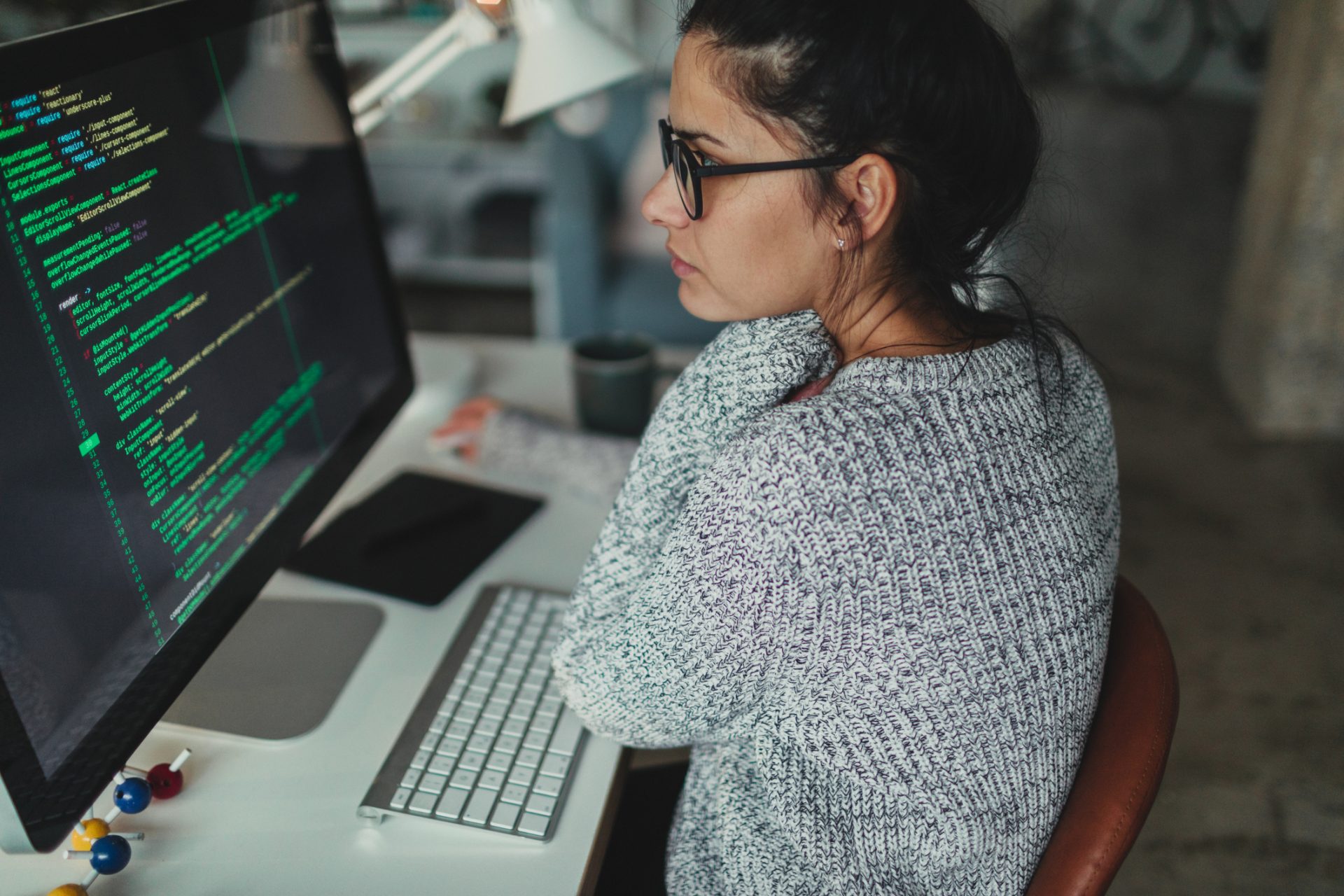
Hi, here the solution for this HackerRank problem “John works at a clothing store. He has a large pile of socks that he must pair by color for sale. Given an array of integers representing the color of each sock, determine how many pairs of socks with matching colors there are.”
For example, there are n = 7 socks with colors ar=[1, 2, 1, 2, 1, 3, 2] . There is one pair of color 1 and one of color 2 . There are three odd socks left, one of each color. The number of pairs is 2.
If this is difficult to understand we can see the arrray like this:
[‘red‘, ‘blue’, red‘ , ‘blue’, ‘red‘, ‘yellow’, ‘blue’] -> So we have just 1 pair of red socks color: 1 pair of red
[‘red’, ‘blue‘, red’ , ‘blue‘, ‘red’, ‘yellow’, ‘blue‘]-> So we have just 1 pair of blue socks color: 1 pair of blue
And finally the total numbers of pair is 2.
Here the Javascript code solution:
// Function that will return the numbers of pairs in the array
function sockMerchant(n, arr) { // parameters
const sortedArr = arr.sort(); // sorting the array will help to check the pairs
let currentPosition = 0; // to save the current position of each item in te array
let nextPosition = 0; // to save the next position of each item in te array
let counter = 0; // this counter will be incremented by 1 in every pair
// To go though every array position
for(let i = 0; i < n - 1 ; i++) { // check that here we are using the "n" parameter instead of array.length (also could work) but use "n"
currentPosition = sortedArr[i]; // holding the current position
nextPosition = sortedArr[i+1]; // holding the next position
// if current position and next positions are the same then this is a pair of "socks"
if (currentPosition === nextPosition) {
counter++; // increment the counter because is a pair
i++; // increment the counter that handler the positions in the array
}
}
return counter;
}
sockMerchant(9, [10, 20, 20, 10, 10, 30, 50, 10, 20])
Result:

By Cristina Rojas.