ES6 – Spread operator in Arrays
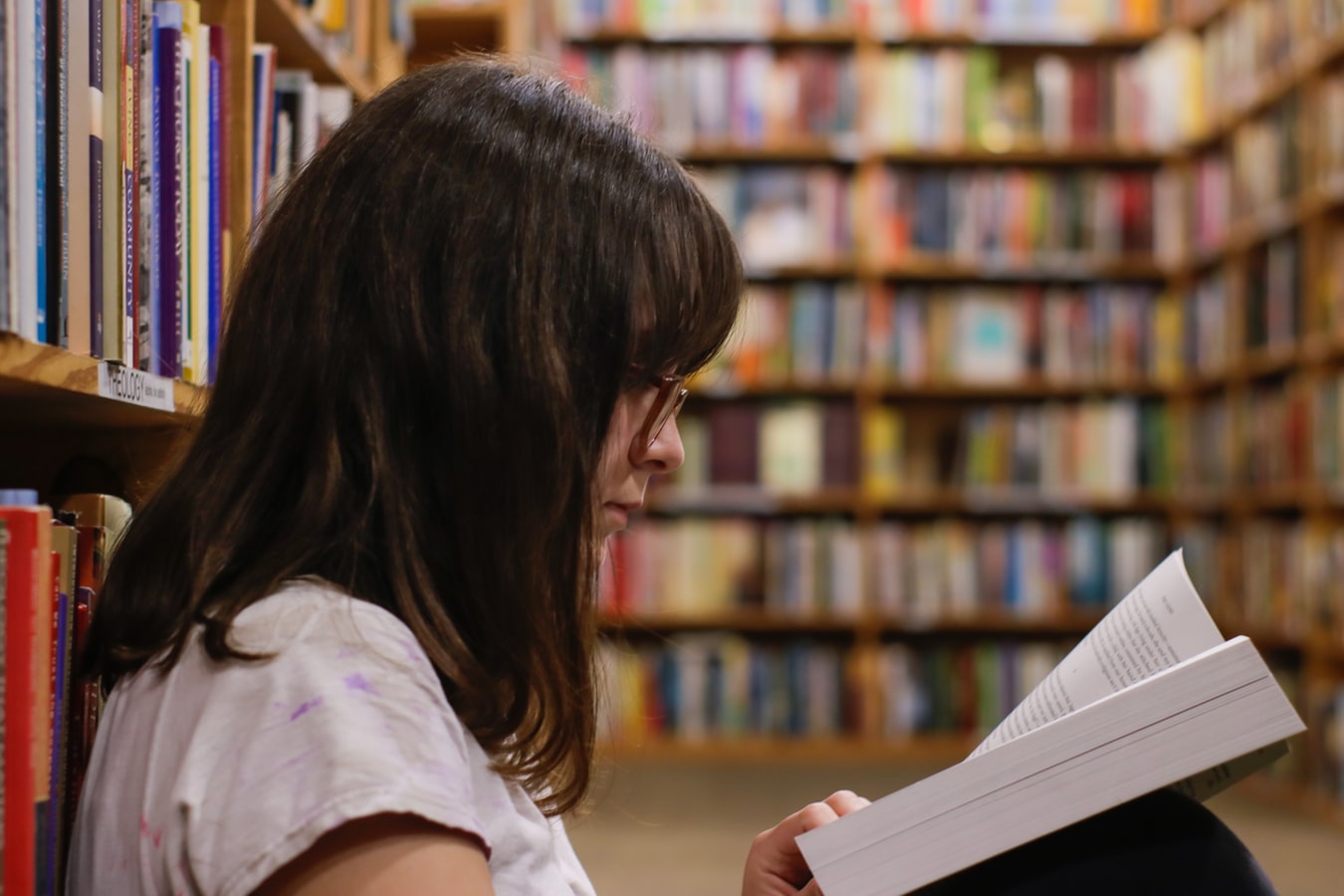
Hi, the spread operator is part of ES6 and is represented by “…” token, spread operator splits an iterable object into individual values.
We can use Spread operator to make Array values a part of another Array.
If we have an Array with values and we want to insert those values into another Array then we can use the Spread operator, look:
Copy values of an Array into another Array
// Arrays with data
const firstArray = [2, 3, 4];
// Inserting the values of the firstArray into the secondArray
const secondArray = [1, ...firstArray, 5];
console.log("secondArray --->", secondArray);
Result:

Push Array values into another
If we want to insert the Array values and the end of the other Array, then we can use the Spread operator like this:
// Arrays with data
const firstArray = [2, 3, 4, 5];
const secondArray = [1];
// Inserting the values of the firstArray into the secondArray
secondArray.push(...firstArray);
console.log("secondArray --->", secondArray);
Result:

Spread Operator in multiple Arrays
We can use Spread operator in multiple Array in just one line, like this:
// Arrays with data
const firstArray = [2, 3, 4, 5];
const secondArray = [1];
const thirdArray = [...secondArray, ...firstArray]; // Joining firstArray and secondArray [1, 2, 3, 4, 5]
const fourArray = [30];
// Function that return a subtraction
const myFunction = (a, u, v, x, y, z) => {
const sum = u + v + x + y + z; // Just sum the value of the parameters
return a - sum; // returning subtraction
};
// Executing function and using spread operator to pass the values of the Arrays
const result = myFunction(...fourArray, ...thirdArray);
console.log("result --->", result);
Result:

By Cristina Rojas.