What is the .bind( ) method in Javascript?
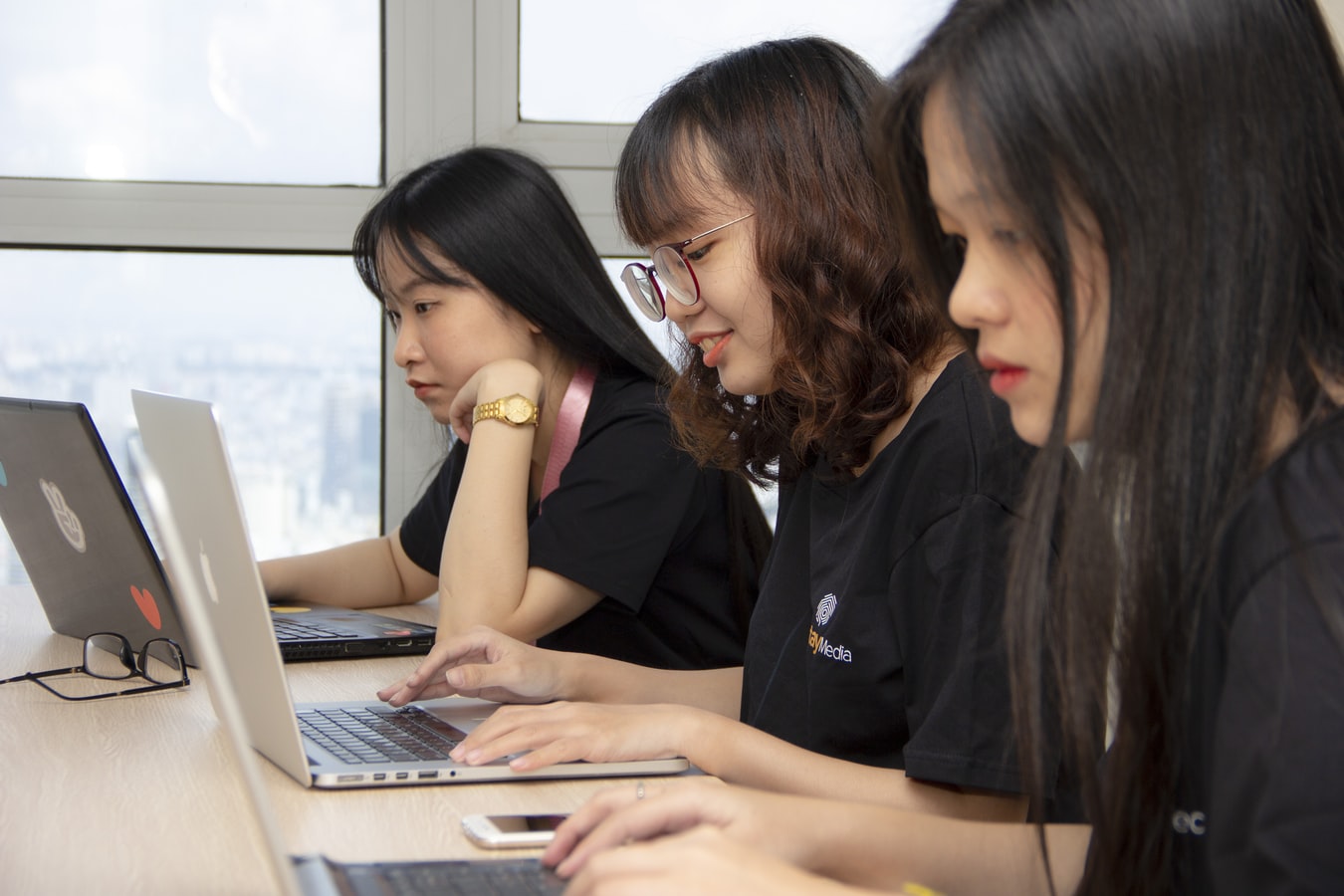
Hi, in this post I’m going to explain what is the .bind() method and how can you use this method in your Js code.
The bind() make a function that, no matter how it is called, is called with a particular “this” value.
Let see this example:
// bind() is to make a function that, no matter how it is called,
// is called with a particular "this" value
const person = {
firstName: "Cristina",
lastName: "Rojas",
fullName: function () {
return this.firstName + " " + this.lastName;
}
}
let getFullName = function() {
console.log('The full name is' + ' ' + this.fullName());
};
// Binding the "this" (context) of "person object"
// to the function "getFullName"
let result = getFullName.bind(person);
result(); // result is the created function
Result:

Look the “result” is declared and the we are assigning the getFullName.bind(person); at that point we are using the .bind() method a new function is created and save it into “result” variable and also is binding the “this” values of the person object (the context of that object).
Nice, now After we use .bind() method we can use the created function just like regular (normal) function it means that we can modify the created function (result) to accept parameters and pass that parameters, look:
const person = {
firstName: "Cristina",
lastName: "Rojas",
fullName: function () {
return this.firstName + " " + this.lastName;
}
}
let getFullName = function(age, profession) {
console.log('The full name is ' + ' ' + this.fullName());
console.log(this.fullName() + ' is ' + age + ' and is a ' + profession)
};
// Binding the "this" (context) of "person object"
// to the function "getFullName"
let result = getFullName.bind(person); // result is the created function
result(31, 'Telematics Engineer'); // result can accept parameters
Result:

See how we are passing 2 parameters (31, ‘Telematics Engineer’) to the created function (result), and use those parameters into the getFullName function.
By Cristina Rojas.