Flatten a structure with Recursion JS
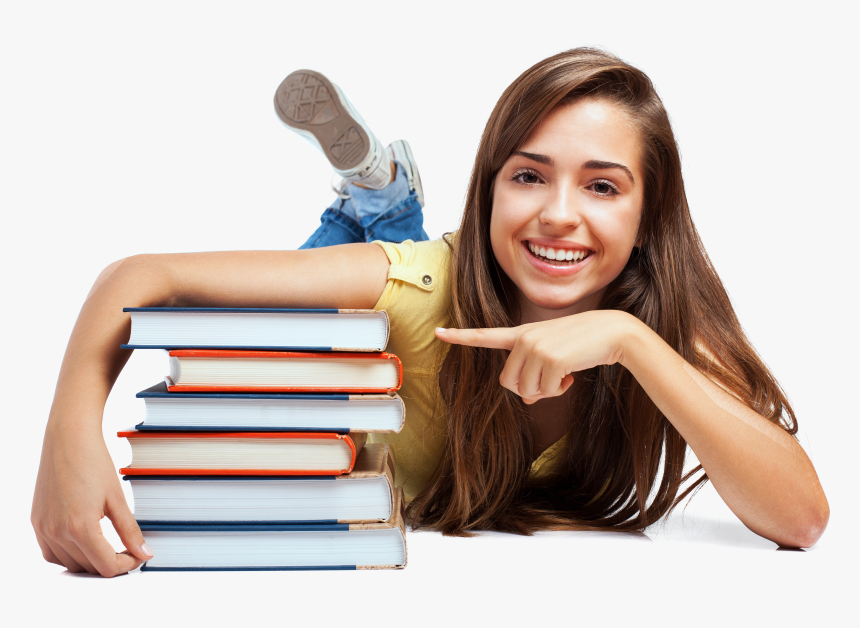
Hi, here a solution of how can we flatten a data structure using recursion.
Given a data structure we need to flatten that and get back the result in this format:
const expected = [
{ value: 'value1' },
{ value: 'value200' },
{ value: 'value3' },
{ value: 'value4' },
{ value: 'value5' }
];
So here the solution:
// Function that flatten a passed data structure
const flatten = (input) => {
let expected = []; // Array to save the final result
// Validating if the input have something
if (input && input.length > 0) {
// Go through every position in that array
input.forEach((item) => {
// saving the item value into the expected array
expected.push({ value: item.value }); // example -> [{ value: value1}]
// Validating again if item.children array have something
if (item.children && item.children.length > 0) {
// expected is keeping the current expected elements and adding the new values that flatten (recursion) give us again
expected = ([...expected, ...flatten(item.children)]);
}
})
}
// returning the expected array
return expected;
}
// Data structure (array of objects)
const data = [
{
value: 'value1',
children: [
{
value: 'value200',
children: [
{
value: 'value3',
children: []
}
]
},
{
value: 'value4',
children: []
}
]
},
{
value: 'value5',
children: [],
}
];
// Inserting into result the return of flatten function
const result = flatten(data);
// Printing the result into the browser console
console.log('result --->', result)
Result:
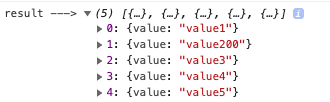
By Cristina Rojas.