What is call() and apply() is Javascript?
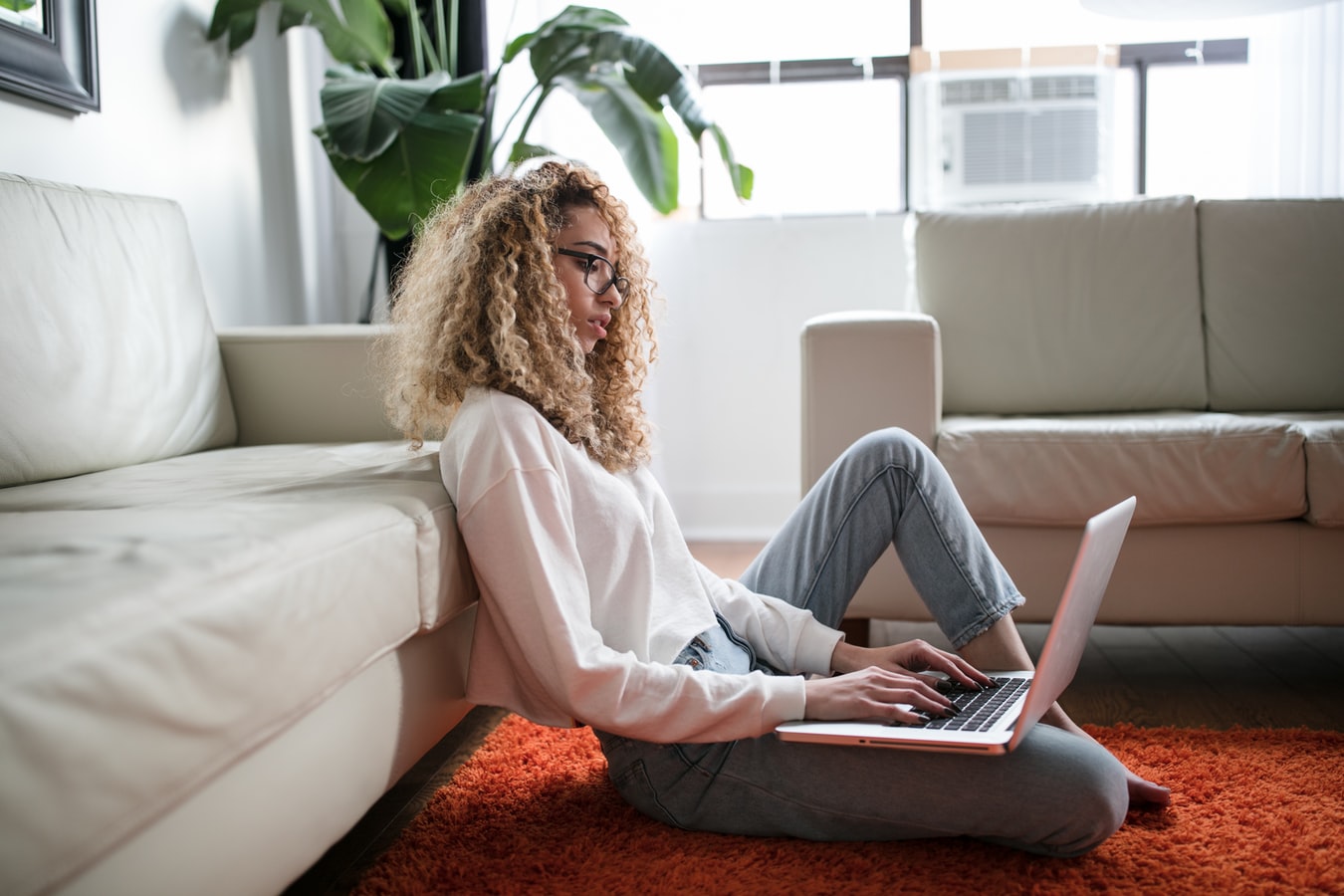
Hi, in this post I’m going to explain the use of call() and apply() methods in Javascript.
The call() method calls a function with a given “this” value and arguments provided in individual way.
If we compare call() and apply() methods – both serve the exact same purpose but the only difference is that call() method expects all parameters to be passed individually, whereas apply() method expects an array of all the parameters.
If we follow the same example of the bind() explanation and instead of bind() we can modify the code by using call() and apply(), look:
// person object
const person = {
firstName: "Cristina",
lastName: "Rojas",
fullName: function () {
return this.firstName + " " + this.lastName;
}
}
let getFullName = function(age, profession) {
console.log(this.fullName() + ' is ' + age + ' and is a ' + profession)
};
// call() expects all parameters to be passed individually
getFullName.call(person, 31, 'Telematics Engineer'); // Executes the function it was called immediately
// apply() method expects an array of all the parameters
getFullName.apply(person, ['31', 'Telematics Engineer']); // Executes the function it was called immediately
Result:

The main differences between bind()
and call()
is that the call()
method:
- Accepts additional parameters as well
- Executes the function it was called upon right away.
- The
call()
method does not make a copy of the function it is being called on.
By Cristina Rojas.