Search for elements in array and modify them
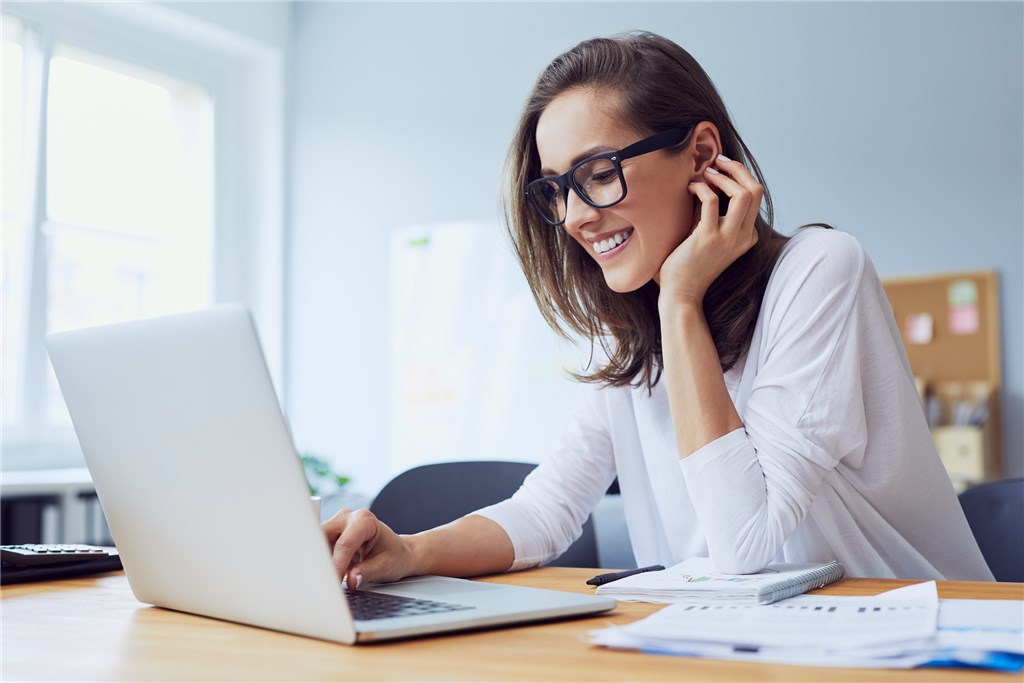
Hi, One of the things that I like to do when I don’t understand something (code or life) is search about the explanation, see how other people solve the problem if is technical, check different solutions for solve that problem and compare different type of methods that can help me with that doubt.
In this case, I’m going to teach you how to “search for elements in arrays” using Javascript code.
Let suppose that we have this array called todoList that save all my “things to do…” like:

Nice, now let suppose that I need to “update, change” the “read book” todo by “go shopping“, how can I search for that element in the array and change that text?
To get the value of an element in the array we need to do array[index] example: todoList[1] result will be “read book” see the code:
// My todo list in an array
let todoList = ["cook", "read book", "laundry"]; // 0, 1, 2 positions in the array
const pos1 = todoList[1];
console.log('pos1 --->', pos1);
Result:

.indexOf()
Said this, now we can use the “indexOf()” Javascript method; that will searches the array for the specified element, and returns its position if that element is founded, and if that specific is not in the array then will return -1 also if the element is present more than once, the indexOf() method returns the position of the first occurence.
So let’s see this example: search a specific element in the array and get the index of that element.
// My todo list in an array
let todoList = ["cook", "read book", "laundry"]; // 0, 1, 2 positions in the array
// Function that search the element in the array
function searchElement(elementOfTheArray, updatedElement) {
// positionFound will be position 1 of the array
const positionFound = todoList.indexOf(elementOfTheArray); // indexOf() method searches the array for the specified item, and returns its position
// todoList[1] = "go shopping"
todoList[positionFound] = updatedElement;
// returning the array
return todoList;
}
// Search for this "read book" element and update the value by "go shopping"
searchElement("read book", "go shopping");
Result:

.map()
Cool, Now another solution will be using .map() Javascript method
// My todo list in an array
let todoList = ["cook", "read book", "laundry"]; // 0, 1, 2 positions in the array
// Function that search the element in the array
function searchElement(elementOfTheArray, updatedElement) {
// .map() creates a new array with the results of calling a function for every array element
// .map() method calls the provided function once for each element in an array, in order
return todoList.map((element) => { // provided function () =>
// element is every element in the array todoList
if (element === elementOfTheArray) { // comparing if the current "element" is equal to the parameter passed "elementOfTheArray"
// if is true
return updatedElement // then in that position will return (insert) the new value
}
// map return that 'return' every element in the array
return element;
});
}
searchElement("read book", "go shopping");
Result:

Nice! if you see both solutions work! but which is best? well, depend of the necessity of the program because if we have the todoList array like this: where the “read book” is repeated
// My todo list in an array
let todoList = ["cook", "read book", "laundry", "read book"]; // 0, 1, 2, 3 positions in the array
A) using indexOf() only will affect the first occurrence:
// Result
["cook", "go shopping", "laundry", "read book"]
B) Using .map() will affect all the elements in the array where the condition is true
// Result
["cook", "go shopping", "laundry", "go shopping"]
.findIndex()
This method is similar to .indexOf() (that search only in primitive types like string, number, or boolean), but with the .findIndex() we can use it if we need the index in arrays with non-primitive types (e.g. objects) or your find condition is more complex than just a value.
// My todo list in an array
let todoList = ["cook", "read book", "laundry"]; // 0, 1, 2 positions in the array
// Function that search the element in the array
function searchElement(elementOfTheArray, updatedElement) {
// findIndex returns the index of the first element in an array that pass a test (provided as a function)
const index = todoList.findIndex((todo) => todo === elementOfTheArray);
// Updating the value with the new one
todoList[index] = updatedElement;
// returning the function
return todoList;
}
searchElement("read book", "go shopping");
Result:

By Cristina Rojas.