What is the difference between forEach and for loop?
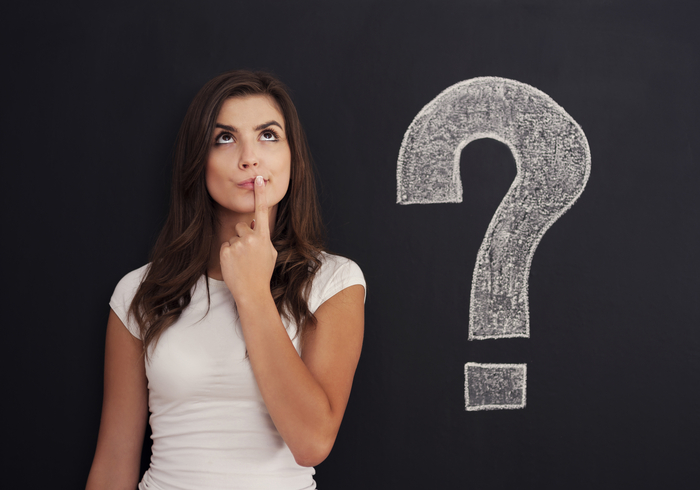
Hi, this is a question that I want to answer because I had the same doubt, so let’s start!
If you have this code with forEach method and for loop the result will be the same:
// Array with some data
const myArray = [1, 2, 3, 4];
// forEach() method
myArray.forEach(function(value) {
console.log('each value --->', value);
});
// for loop
for (var i = 0; i < myArray.length; i++) {
console.log('each value --->', myArray[i]);
}
Result:
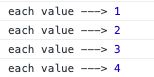
Hmm…the result is the same…but which is better for loop or forEach method?
- forEach is a method of the Array prototype and the “for” loop is not.
- forEach method go through every element in the array and passes it to callback function to every element in the array and “for” loop do not pass a callback function.
- The “for” loop it is not necessarily something that you need to use just in arrays, what I mean is that you can use “for” loop for others thing where you can iterate, look this example:
// Variable with a total value of 5
let total = 5;
// for loop
for (var i = 1; i <= total; i++) {
console.log('number --->', i);
}
Result:
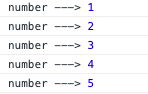
- “for” loop is slightly better because there is not function call for each element involved.
- The forEach method is much easier to use because you don’t need to get frustrated with the counters and validations (i < array.length, i++, etc.) so, Javascript does it for you.
- In “for” loop we can use the keyword “break” if you need to exit from the loop.
- forEach have this parameters (iterator, Index of item, entire array) and “for” loop (iterator, counter, increasing)
Nice, so next time if somebody ask you this question in interview you can answer the points that already mentioned.
By Cristina Rojas.