Simple exercise that show a Queue in Javascript
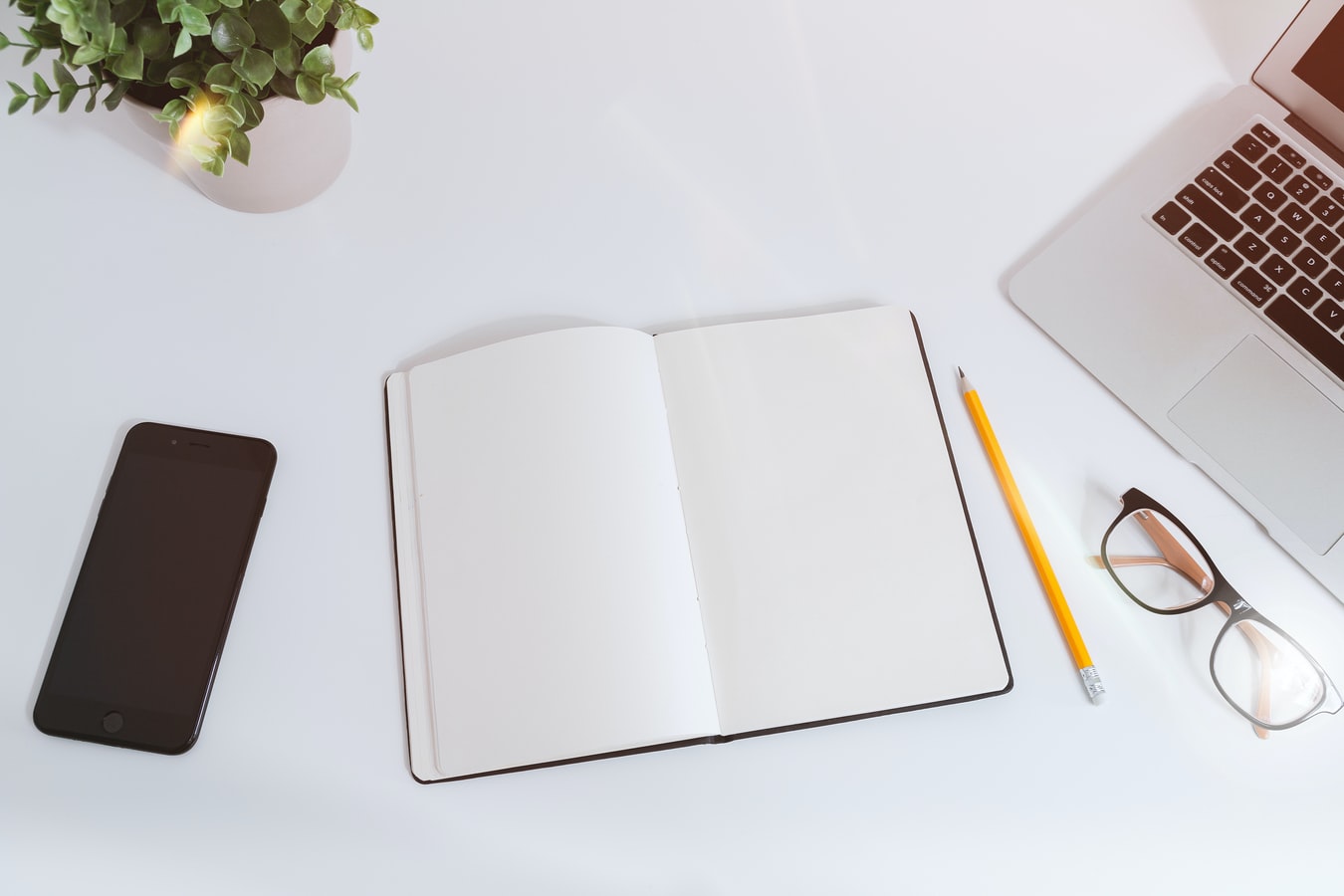
Create a queue data structure. The queue should be a class with methods ‘add’ and ‘remove’.Adding to the queue should store an element until it is removed
Examples
const q = new Queue();
q.add(30);
q.remove(); // 28
Solution
class Queue {
constructor() {
this.data = [50, 20, 28];
}
// Add method
add(item) {
this.data.unshift(item); // .unshift() add the item at the begging of the array pos 0
}
// Remove method
// remove from the Queue - removing First item that was added
remove() {
return this.data.pop(); // .pop() remove the last position of the array
}
}
const q = new Queue();
q.add(30);
q.remove();
console.log('q --->', q)
Result:

By Cristina Rojas.