Multiple conditions in the short circuit
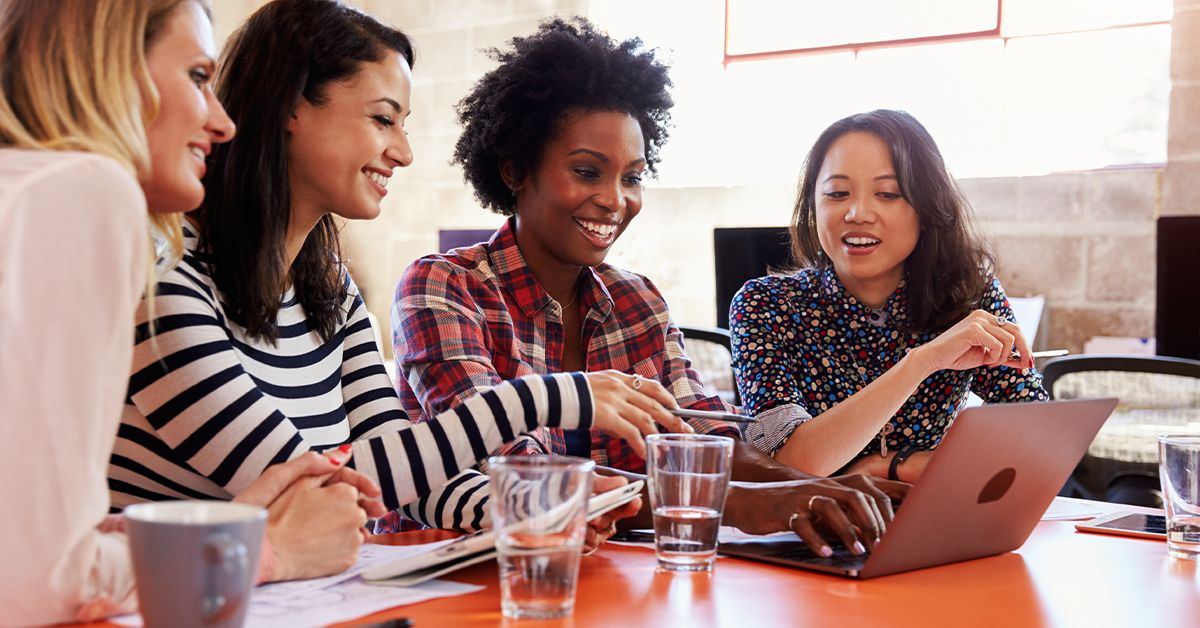
Hi, this post is the continuation of the What is short circuit condition? and how works in React so let’s see an example of multiple conditions in the short circuit condition.
We can insert multiple conditions with the && (and) operator to rendering or not:
// Dependencies
import React, { useState } from 'react'
// Types
import { FriendsType } from './../types/types'
// MUI
import { Box, Button } from '@material-ui/core'
// Styles
import useStyles from './MyList.styles'
// Interface
interface Props {
data: FriendsType[]
}
const MyList = ({ data }: Props) => {
// Local state
const [showList, setShowList] = useState(false) // The value of show is false
const isFernandoFriend = false
// Style
const classes = useStyles()
return (
<Box className={classes.mainContainer}>
<Button
variant="contained"
color="primary"
{/* Everytime that I click the button I'm changing the showList value to true or false */}
onClick={() => {
setShowList(!showList)
}}
>
{/* If showList is true then show 'Hide List' text */}
{/* If showList is false then show 'Show List' text */}
{showList ? 'Hide List' : 'Show List'}
</Button>
<Box>
{/* showList have a true value && (and) also isFernandoFriend have a true value? */}
{/* YES! both have a true value, then ---> rendering! */}
{/* NO! one of this variables have a false value ---> Don't rendering!, never rendering! */}
{showList && isFernandoFriend && (
<ul className={classes.list}>
{data.map((friend, index) => {
return <li key={`friend-${index}`}>{friend.name}</li>
})}
</ul>
)}
</Box>
</Box>
)
}
export default MyList
The result from the example above will be: “The component will never be rendered again” so if we click the button we will not see nothing in the browser:
First click
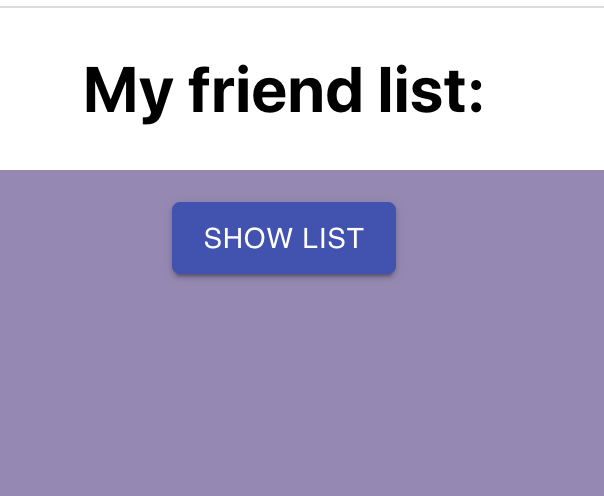
Second click
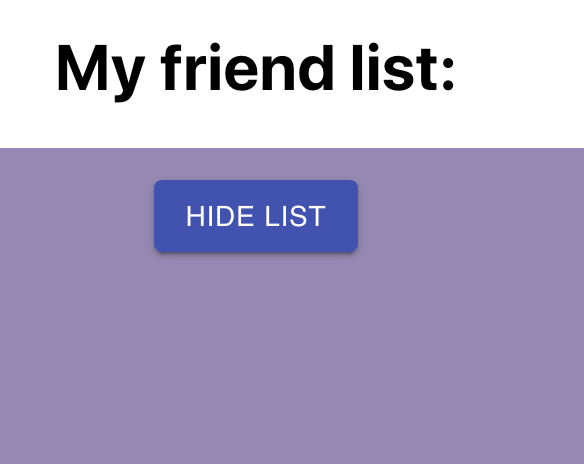
We can insert multiple conditions with the || (or) operator to rendering or not:
// Dependencies
import React, { useState } from 'react'
// Types
import { FriendsType } from './../types/types'
// MUI
import { Box, Button } from '@material-ui/core'
// Styles
import useStyles from './MyList.styles'
// Interface
interface Props {
data: FriendsType[]
}
const MyList = ({ data }: Props) => {
// Local state
const [showList, setShowList] = useState(false) // The value of show is false
const isFernandoFriend = false
const isAlmaFriend = true
// Style
const classes = useStyles()
return (
<Box className={classes.mainContainer}>
<Button
variant="contained"
color="primary"
{/* Everytime that I click the button I'm changing the showList value to true or false */}
onClick={() => {
setShowList(!showList)
}}
>
{/* If showList is true then show 'Hide List' text */}
{/* If showList is false then show 'Show List' text */}
{showList ? 'Hide List' : 'Show List'}
</Button>
<Box>
{/* showList have a true value? || (or) isFernandoFriend have a true value? || (or) isAlmaFriend have a true value? */}
{/* YES! if one of this variables have a true value, then ---> rendering! */}
{/* NO! if all of this variables have a false value ---> Don't rendering!, never rendering! */}
{(showList || isFernandoFriend || isAlmaFriend) && (
<ul className={classes.list}>
{data.map((friend, index) => {
return <li key={`friend-${index}`}>{friend.name}</li>
})}
</ul>
)}
</Box>
</Box>
)
}
export default MyList
The result from the example above will be: “The component is rendering because isAlmaFriend variable have a true value:
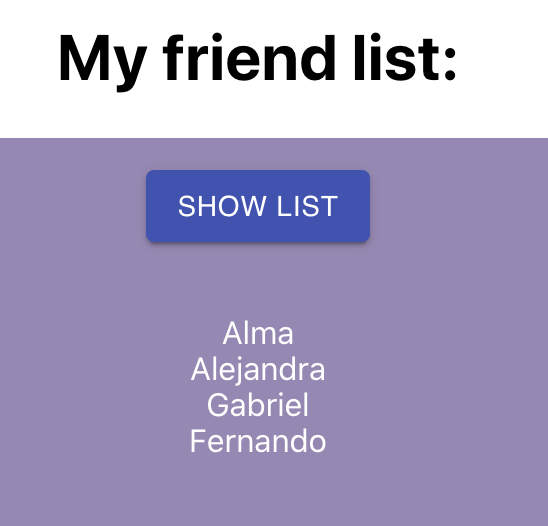
By Cristina Rojas.