ES6 – The return & throw methods in a generator function
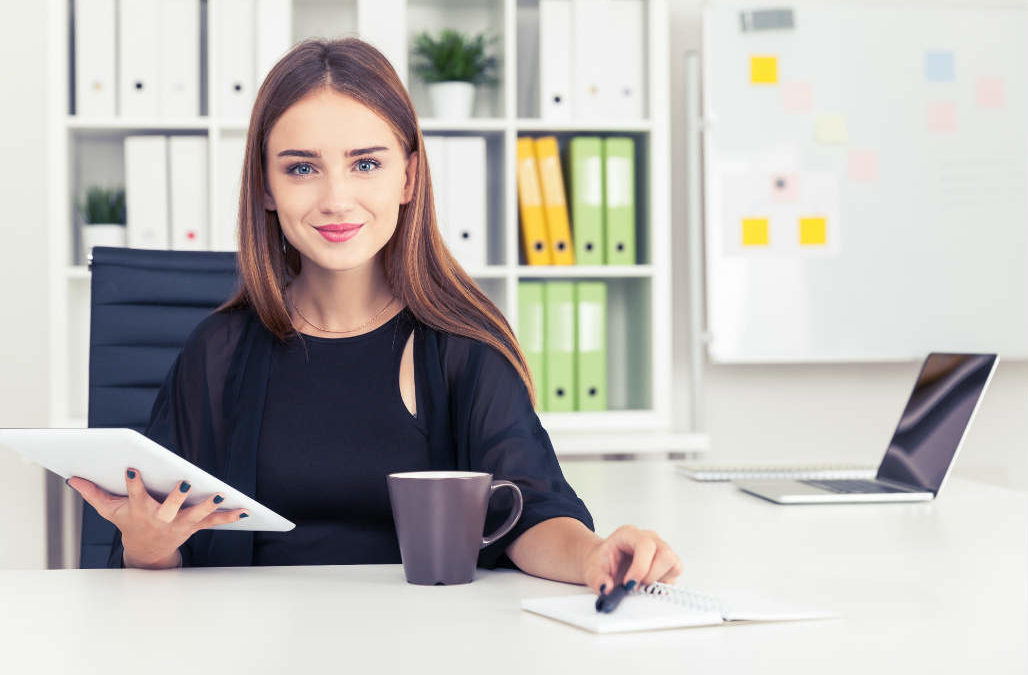
Hi, every time that we need to end a generator function before it has yielded all the values we can use the return( ) method that if of the generator object.
so, the return() method takes an optional argument, representing the final value to return, check this next examle:
function* my_generator_function() {
yield 100;
yield 200;
yield 300;
}
let generator = my_generator_function();
console.log("value --->", generator.next().value);
console.log("value --->", generator.return(500).value);
console.log("value --->", generator.next().done);
Result:
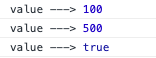
The throw() method
We can pass an exception to the throw() method that we want to throw.
function* my_generator_function() {
try {
yield 100;
} catch (e) {
console.log("1 Exception");
}
try {
yield 200;
} catch (e) {
console.log("2 Exception");
}
}
let generator = my_generator_function();
console.log("value --->", generator.next().value);
console.log("value --->", generator.throw("exception string").value);
console.log("value --->", generator.throw("exception string").done);
Result:
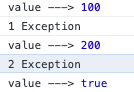
By Cristina Rojas.