Check if an array have duplicated elements Js
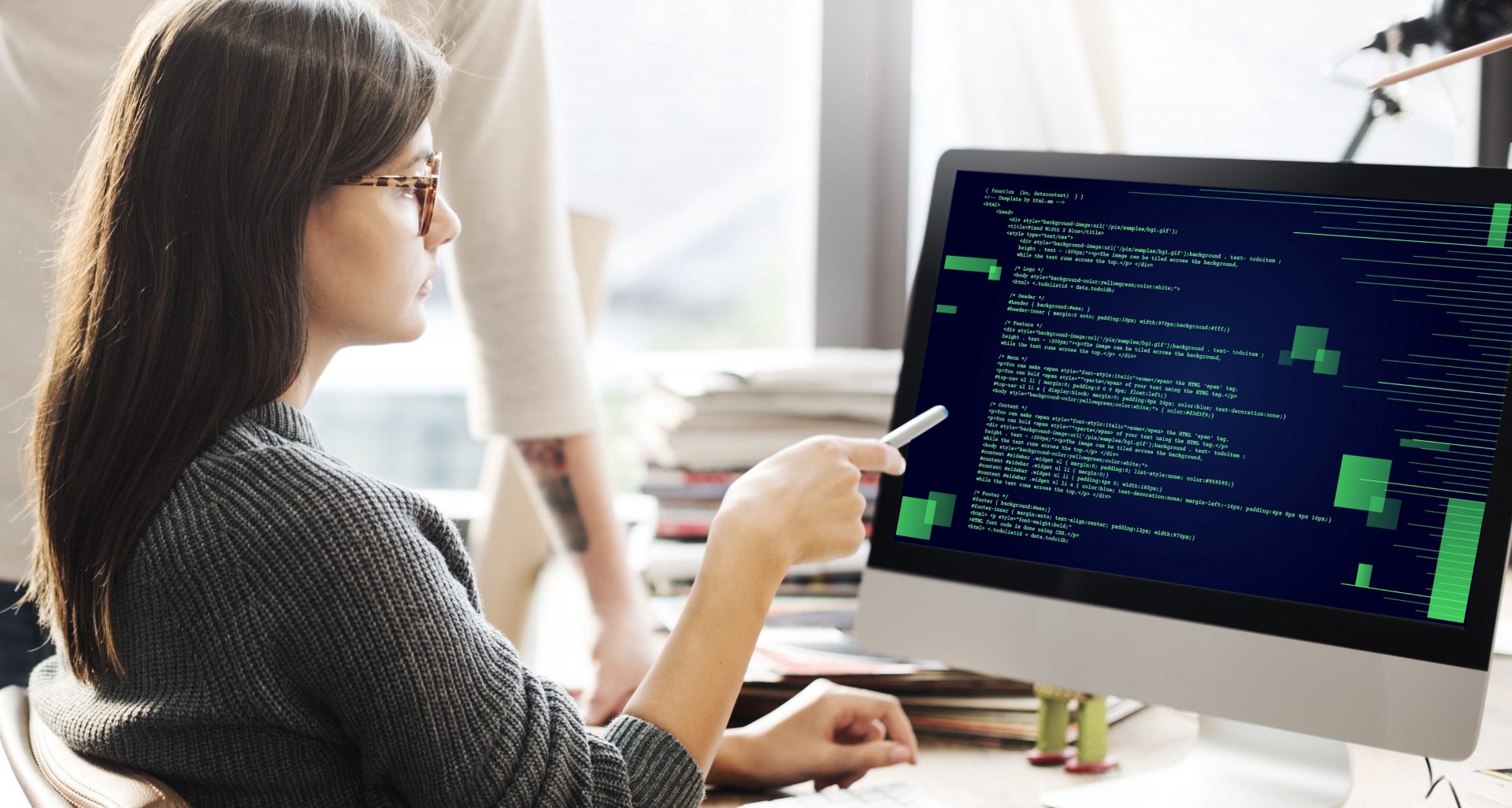
Hi, here some code solutions that will help us to check if an array have duplicated elements or not.
First Solution
function checkForDuplicates(array) {
// To save all the values that are not repited
let helperArray = [];
for (let i = 0; i < array.length; i++) { // To go through every elemebt of the array [1, 3, 5, 4, 5]
// If the helperArray DOES NOT have that "value"
if (helperArray.indexOf(array[i]) !== -1) { // .indexOf("theVALUE") -> will return the position of this value if is in the array
// -1 means that the element is not in te array
// and because we are denying then means that "if that element it is in the array" then return 'Yes values repited!'
return `Yes Values repited, and the value repited is: ${array[i]}` // or return true
}
// The helperArray is saving every element that is not repited
helperArray.push(array[i])
}
return false
}
checkForDuplicates([1, 3, 5, 4, 5]);
Result:

Second Solution
function checkForDuplicates(array) {
// To save all the values that are not repited
let helperArray = [];
for (let i = 0; i < array.length; i++) { // To go through every elemebt of the array [1, 3, 5, 4, 5]
// If the helperArray Yes includes this value
if (helperArray.includes(array[i])) {
return `Yes Values repited, and the value repited is: ${array[i]}` // or return true
}
// If helperArray does not include that value then save the value into helperArray
helperArray.push(array[i])
}
return false
}
checkForDuplicates([1, 3, 5, 4, 3]);
Result:

Third solution:
let myArray = [1, 3, 5, 4];
let hasDuplicate = myArray.some((val, i) => myArray.indexOf(val) !== i);
console.log('hasDuplicate---->', hasDuplicate)
Result:

Four Solution:
function checkForDuplicates(array) {
return new Set(array).size !== array.length
}
checkForDuplicates([1, 3, 5, 4, 1]);
Result:
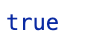
By Cristina Rojas.