Dimensional Arrays explanation
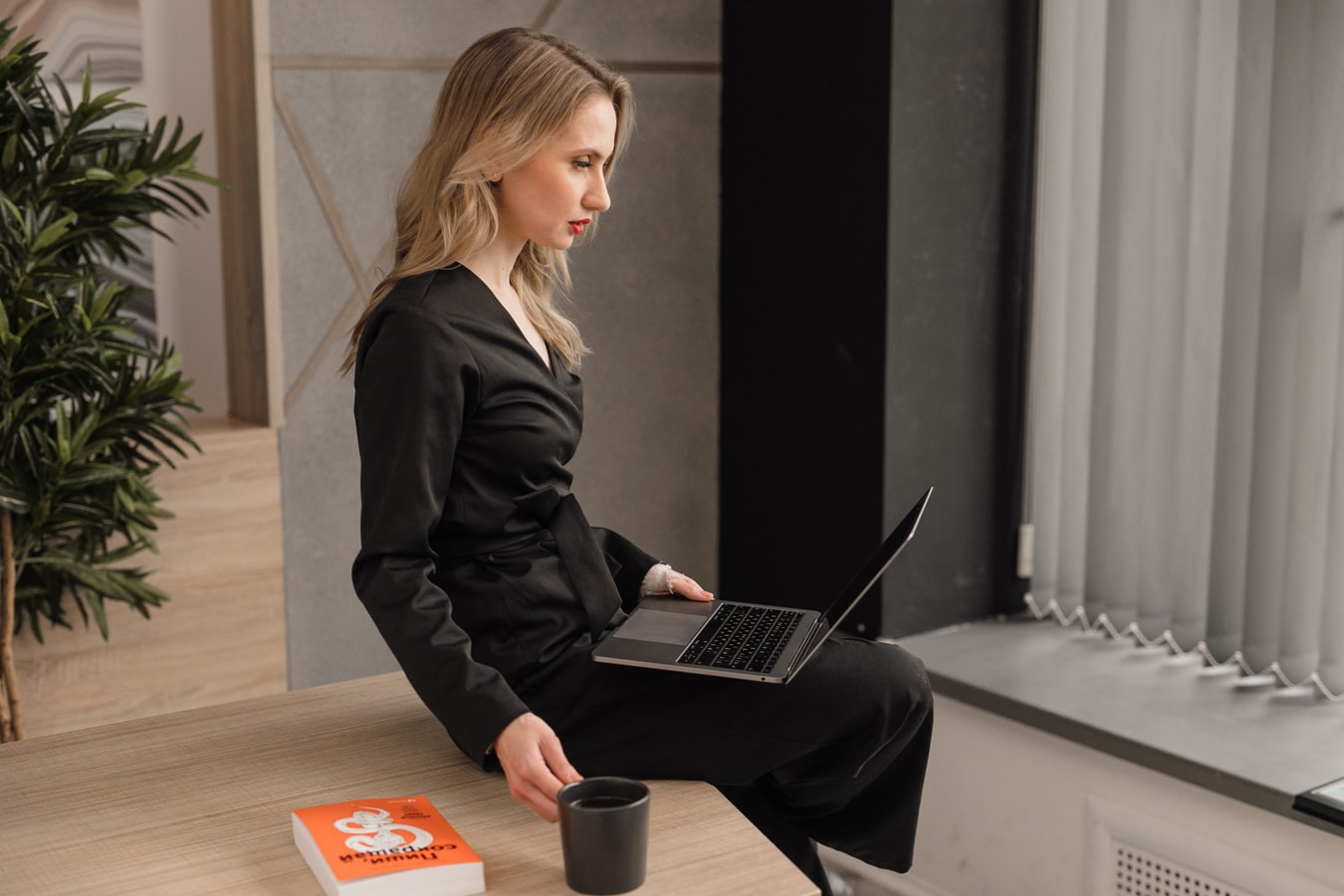
Hi, for a long time I had many doubts about the term of dimensional array and studying this terminologies I learn about it and I want to share with you the explanation of the dimensional arrays, so don’t worry I will explain you this in a very easy way to understand 🙂
Ok, an Array is represented as a pair of square brackets: [ ] and is a storage where we can save a lot of things like strings, numbers, functions, operations, other arrays, everything you want like this:
let myArray = [function () { console.log('hi!')}, 3, 'yes', 20 + 20, ['cristina']];
The view of this array in the browser console will be this:

Nice! so, an array can save other arrays? Yes! yes it can!
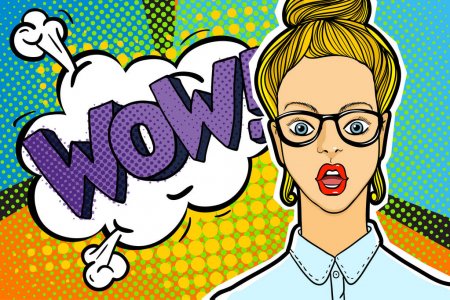
Ok, if an array save other arrays, then this is named as a multidimensional array or matrix.
For example:
A 2 Dimensional (2D) array will be like this
let mainArray = [['cristina'], ['hi'], ['welcome']];
but, why? well a 2 dimensional array is a structure like a spreadsheet with rows and columns, example:
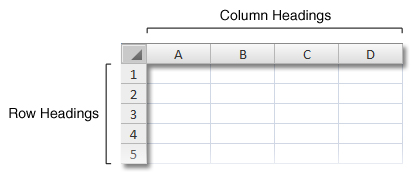
So, back to our code, a better way to understand our 2 dimensional array will be break our array in this format:
let mainArray = [ // 1 column
['cristina'], // 1 row
['hi'], // 2 row
['welcome'] // 3 row
];
In this case we have 3 rows and 1 column, where every row is each item of the mainArray and every column is the item of the child array.
Nice, ok let’s see other example:
let mainArray = [['cristina', 'rojas'], ['hi', 'there'], ['welcome', 'to learntechsystems']];
If we formatting the mainArray then will have this:
let mainArray = [ // 1 column, 2 column
['cristina', 'rojas'], // 1 row
['hi', 'there'], // 2 row
['welcome', 'to learntechsystems'] // 3 row
];
In this case we have 3 rows and 2 columns.
Cool, at this point we have a square matrix because a square matrix is a matrix with the same number of rows and columns.
Here an image just for reference about dimensions:
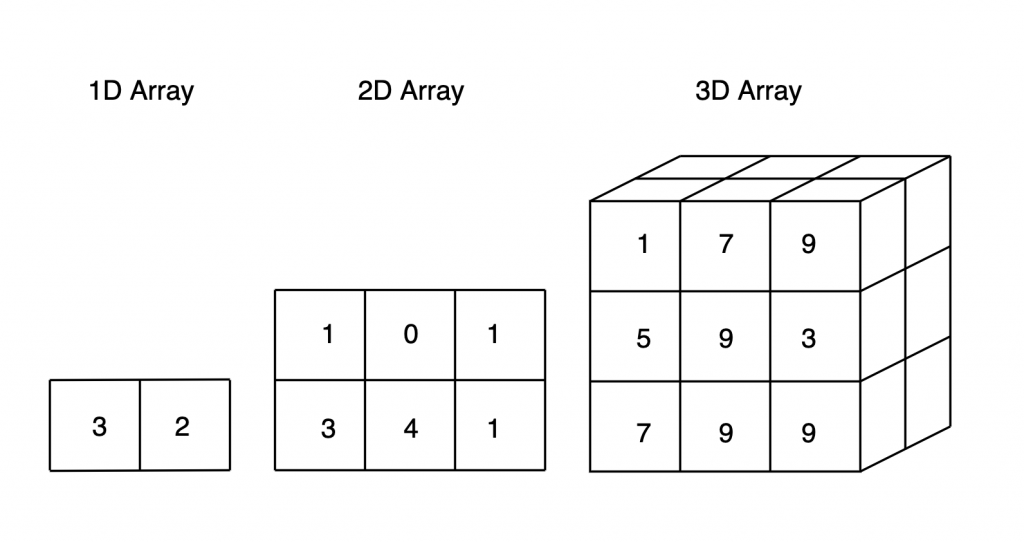
1D Array
let mainArray = ['3', '2']; // linear array
2D Array:
let mainArray = [ // 1 c, 2 c, 3 column
['1', '0', '1'], // 1 row
['3', '4', '1'] // 2 row
];
3D Array:
let mainArray = [ // 1 column 2 column 3 column
[ ['1', 'x'], ['7', 'y' ], ['9', 'z'] ], // 1 row
[ ['5', 'a'], ['9', 'b'], ['3', 'c'] ], // 2 row
[ ['7', 'r'], ['9', 's'], ['9', 't'] ] // 3 row
];
Nice! in the next post you will lear how to create dimensional arrays and and how to initialize them.
By Cristina Rojas.