What is a Callback in Javascript?
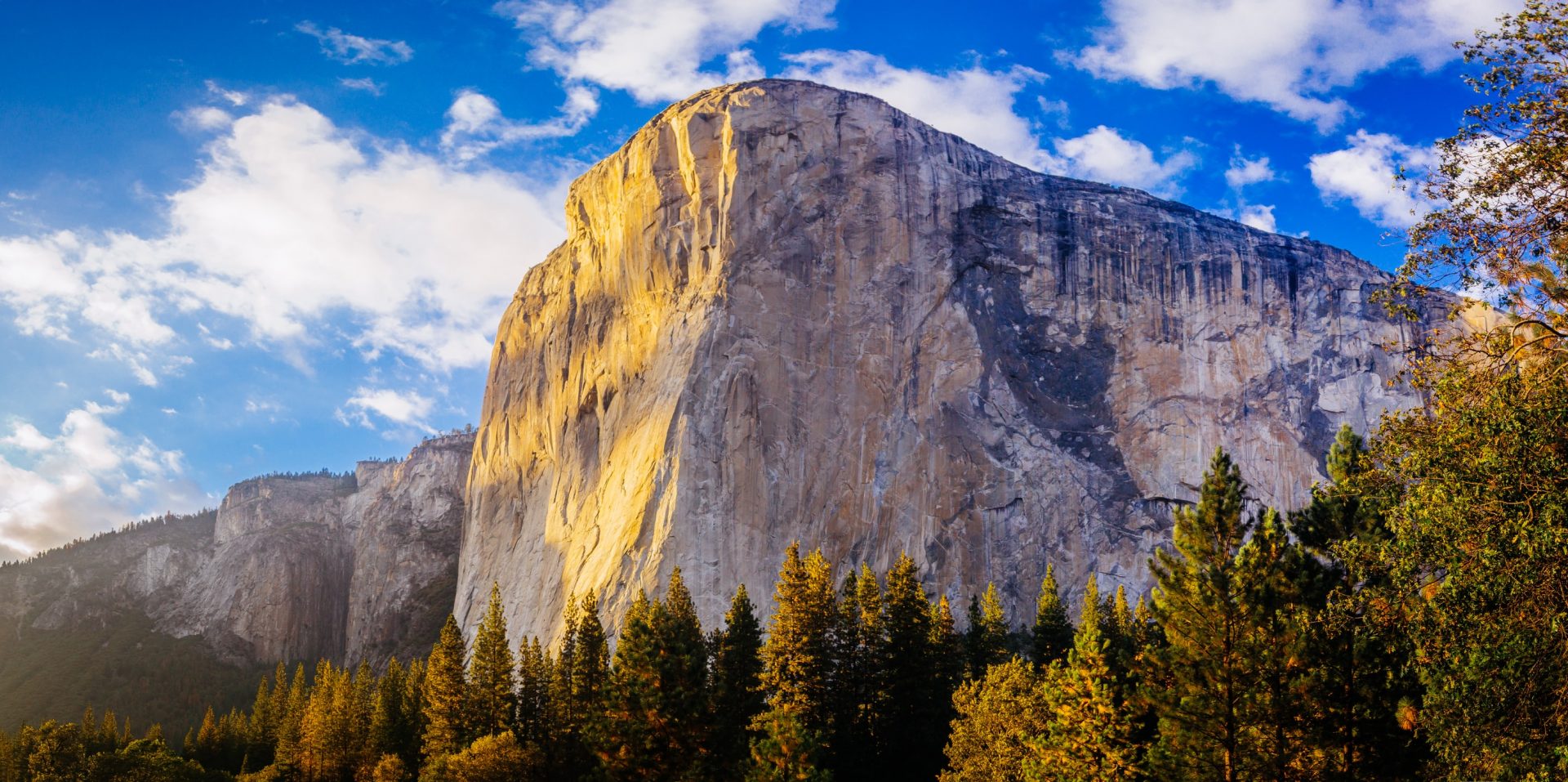
Hi, by definition a Callback is a function that is to be executed after another function has finished executing, hence the name “call back” and callbacks are great way to handle something after something else has been completed.
Let’s see a clear example of Callback:
// Let suppose that this is our database
let users = ['Cristina', 'Carlos', 'Gonzalo'];
// Function to add a user into the database
function addUser(username) {
setTimeout(function() {
users.push(username); // Adding the user to the database
}, 200); // Let's suppose that this is a 3 seconds delay because our database is in other country
}
// Function that show all the users that are in our database
function getUsers() {
setTimeout(function() {
console.log('users ->', users);
}, 100); // 1 second as delay
}
// 1
addUser('Karolina'); // Adding a new user to our database
// 2
getUsers(); // Show all the users that are in our database
Result:

If you see addUser(‘Karolina’) is declared first and then getUsers(), but getUsers() is executed first because the delay is less than than the function addUser().
This is the reason that we see only 3 users and not 4 users is because our function addUser() is executed after getUsers() has been executed.
But we can remedy this enforcing the order of our operations, and here is a good example of how the callback come to solve this situation, so let’s see the same example but with the Callback:
// Let suppose that this is our database
let users = ['Cristina', 'Carlos', 'Gonzalo'];
// Function to add a user into the database
function addUser(username, callback) {
setTimeout(function() {
users.push(username); // Adding the user to the database
callback(); // after adding a user the funtion getUsers() will be executed
}, 200);
}
// Function that show all the users that are in our database
function getUsers() {
setTimeout(function() {
console.log('users ->', users);
}, 100);
}
// 1
addUser('Karolina', getUsers); // get the call addUser and pass getUsers function as a parameter
Result:

So, in this case because we are passing the function getUsers as a parameter of addUser function, the function addUser() will take the parameter getUsers and executed after the push happened into the database (Array).
So the function addUser can take 2 seconds or 1 hours, etc. but until this function happen then we are going to execute a function that we passed as a parameter that is getUsers and doesn’t matter if this function is faster it does not run until it has been called.
So with this we are try to enforce the order of the operations for execution.
Note: addUser(‘Karolina’, getUsers) at this point we are passing the hole function getUsers and NOT calling the function like this:
addUser('Karolina', getUsers());
By Cristina Rojas.