Render a list in separate block of code with React
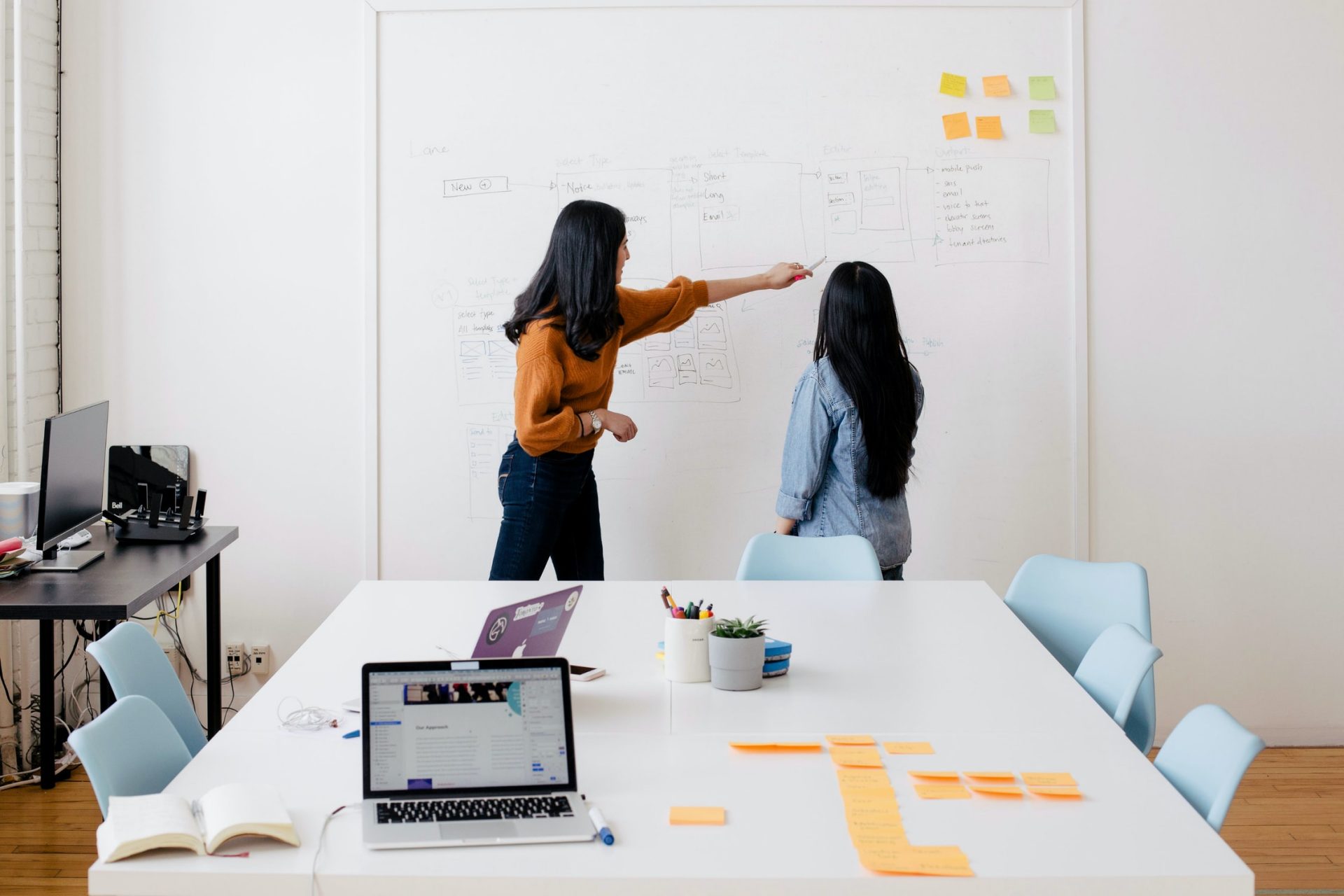
Hi, here an example of how we can separate the HTML that we want to render inside the main component, this is a clean way to work with React components.
Example:
const Uploader = () => {
// My data
const myData = [
{
pruduct: {
name: 'Im the product 1',
description: 'Im the description 1'
}
},
{
pruduct: {
name: 'Im the product 2',
description: 'Im the description 2'
}
},
{
pruduct: {
name: 'Im the product 3',
description: 'Im the description 3'
}
}
]
// Separate code block
const listProducts = myData.map((product, i) => (
<li key={`product-${i}`}>{product.pruduct.name}</li>
))
return (
<section className={styles.mainContainer}>
<ul>{listProducts}</ul>
</section>
)
}
export default Uploader
Result:
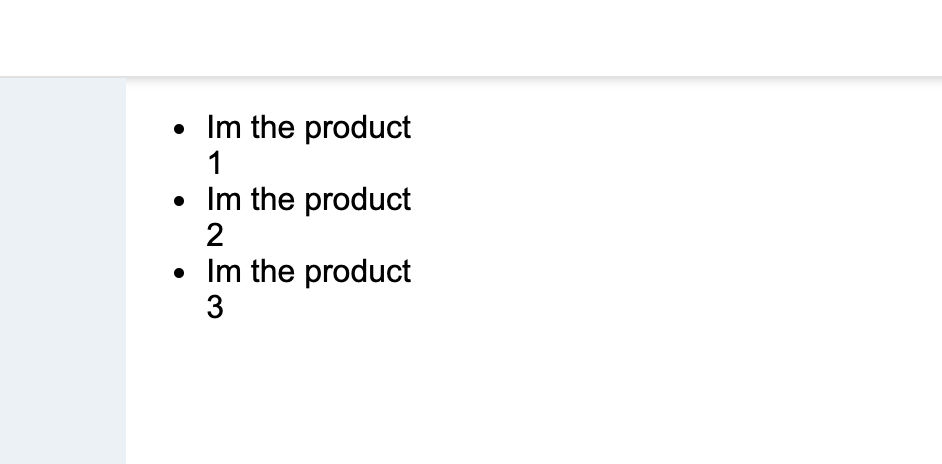
We can separate our HTML code or logic in other pieces of codes as we want, in that way the structure of our main component could be more readable.
By Cristina Rojas.