Javascript reduce method
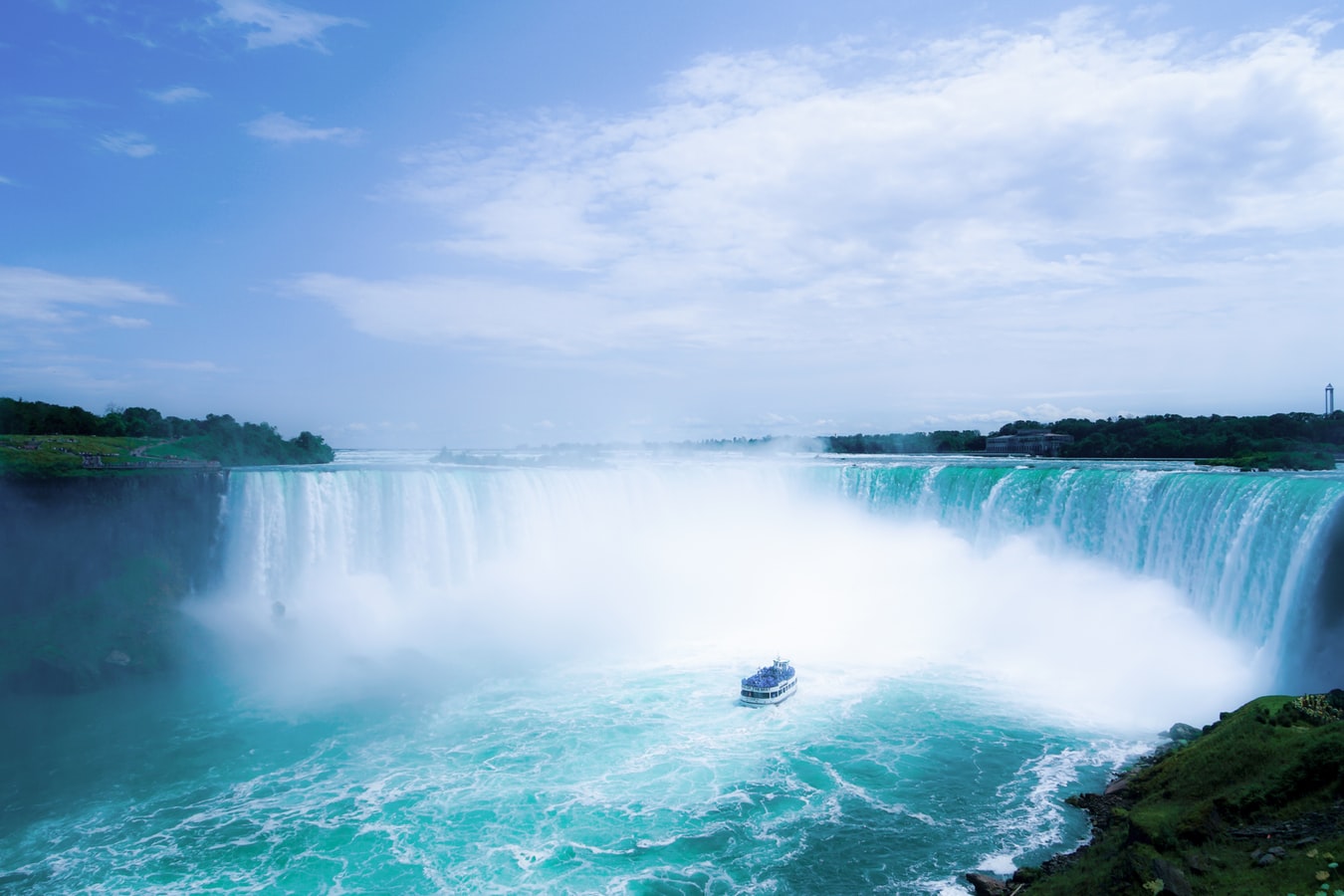
Hi, is important to know how the reduce method works because in that way we can solve some task with less effort.
The reduce method
The reduce method is for handle arrays, and cycles through each number in the array much like it would in a for-loop.
// The array
const myArray = [5, 10, 5, 30];
The reduce method takes 2 parameters:
myArray.reduce( reducerFunction, initialValue )
The reducer function
The reduce method execute a reducer function (that is a callback function) on each element of the array, resulting a single output value.
// reducer function
const sum = myArray.reduce(() => { } );
Note
Don’t be confused:
- this is the reduce method: myArray.reduce()
- this is the .reducer function that we provide () => { }
The reducer function can take 4 arguments (total, currentValue, index, src) => { } you can choose which argument use according to your solution:
1.Total or also called Accumulator (acc).
// The array
const myArray = [5, 10, 5, 30];
When the loop starts the total value is the first number from the left side on the array.
// The array
const myArray = [5, 10, 5, 30];
myArray.reduce((total) =>{ }); // total is 5
2. Current value (cur), amount.
The currentValue is the one next item of the array.
// The array
const myArray = [5, 10, 5, 30];
myArray.reduce((total, currentValue) => { }) // currentValue is 10
3. Current index or idx
Will be the index of the current element in the array.
myArray.reduce((total, currentValue, index) => { }) // current index
4. Source Array or src
Src is the array reduce() was called upon
// The array
const myArray = [5, 10, 5, 30];
myArray.reduce((total, currentValue, index, myArray) => { })
The initial value
This is the second argument of the reduce method, what means that can initialize the total parameter of the reducer function.
myArray.reduce(() => { }, initialValue);
Example:
// The array
const myArray = [5, 10, 5, 30];
const sum = myArray.reduce((total, currentValue) => {
return total + currentValue
}, 5);
console.log('sum -->', sum)
Result:

If we don’t set initial value then the initial value will be the first the value of the total parameter.
myArray.reduce(() => { });
Example:
// The array
const myArray = [5, 10, 5, 30];
const sum = myArray.reduce((total, currentValue) => {
return total + currentValue
});
console.log('sum -->', sum)
Result:

Or this way will be the same as not initialization
myArray.reduce(() => { }, 0);
Example:
// The array
const myArray = [5, 10, 5, 30];
const sum = myArray.reduce((total, currentValue) => {
return total + currentValue
}, 0);
console.log('sum -->', sum)
Result:

By Cristina Rojas.