ES6 – Arrow functions
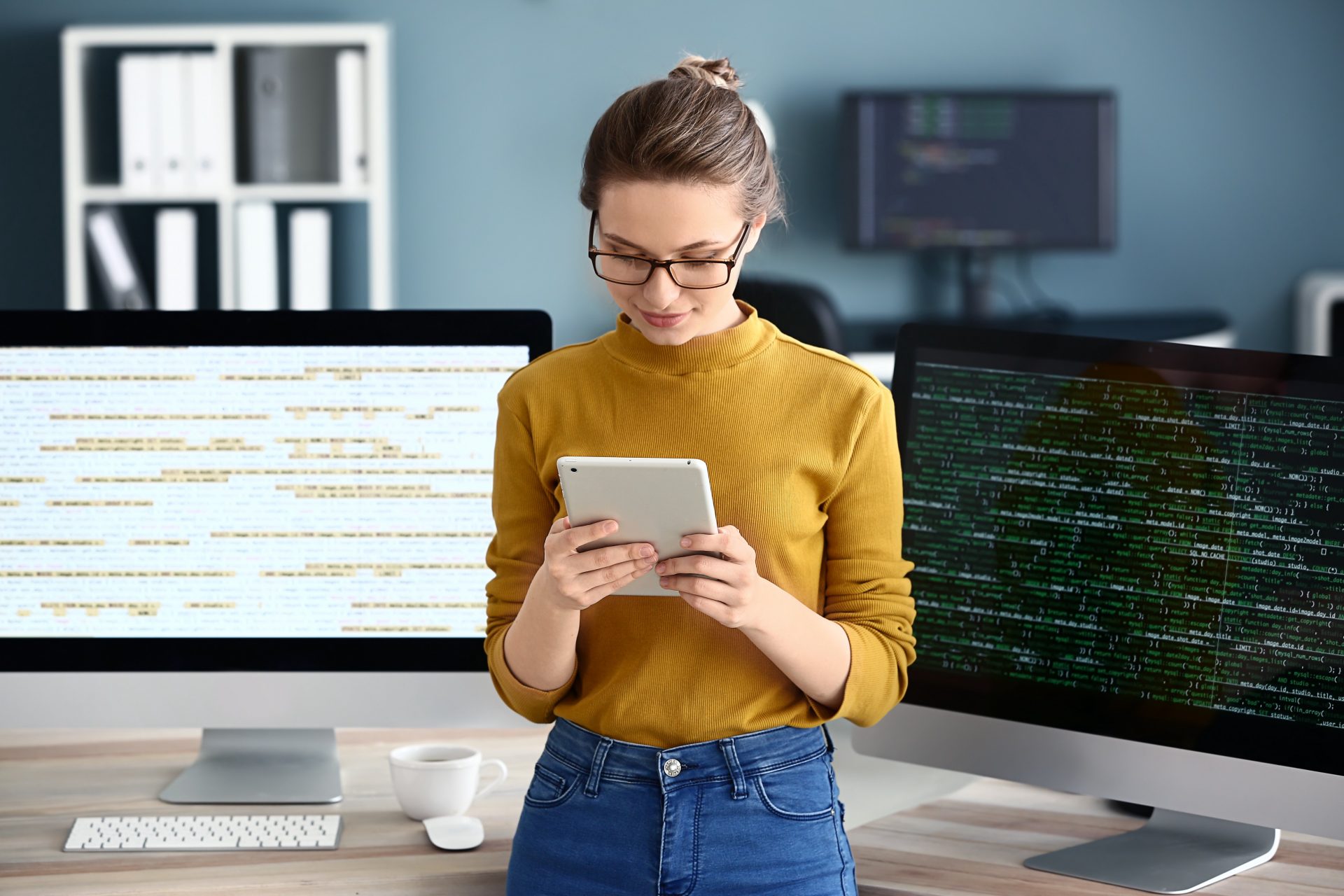
Hi, in this post I’m going to teach you what is an arrow function.
The new way to create functions in ES6 is called “Arrow functions” and we can create arrow functions using the => operator that looks like an arrow 🙂
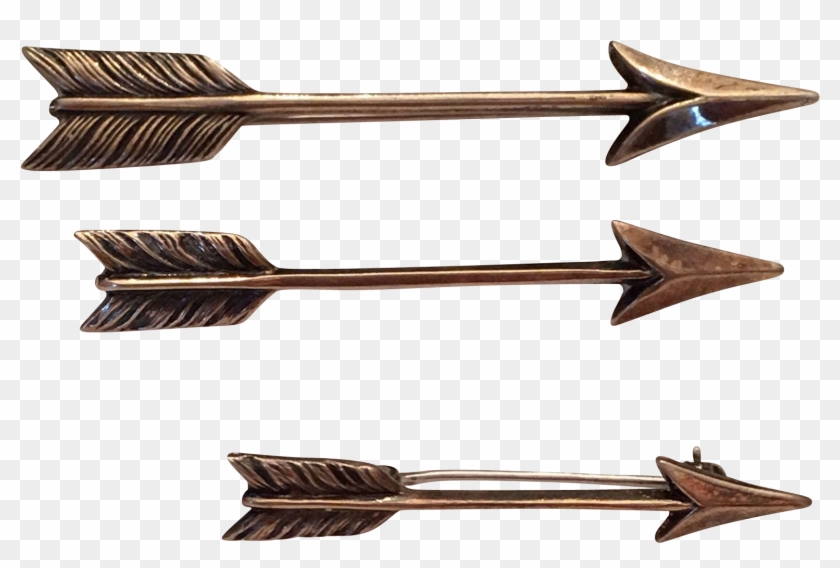
So, one of the advantages of write arrow functions is that the syntax is shorter and other highlight is that arrow functions are anonymous functions (no named functions).
Creating arrow functions
So, this is the format that an arrow function need to have
( ) = > { } // for multiple lines of code
( ) => code here // for 1 line of code, we don’t specify a return because JS does it for us
Example:
Multiple lines of code
// Declaring arrow functions
let myFunction = (a, b) => {
// { } for multiple lines of code
let sum = a + b;
return sum;
};
// Calling myFunction
let result = myFunction(300, 100);
console.log("result --->", result);
Result:

Example:
Single line of code
// Declaring arrow functions
let myFunction = (a, b) => a + b;
// Calling myFunction
let result = myFunction(300, 100);
console.log("result --->", result);
Result: you can only this “single line” way when you have just 1 line of code.

Nice, and finally if we have only 1 parameter in our arrow function then we can delete the pair of parenthesis ( ) and this will perfectly fine.
// Declaring arrow functions
let myFunction = name => name + " rojas";
// Calling myFunction
let result = myFunction("cristina");
console.log("result --->", result);
Result:

Cool, so as I said “one of the advantages of write arrow functions is that the syntax is shorter and other highlight is that arrow functions are anonymous functions (no named functions).”
By Cristina Rojas.