Creating Two-dimensional array
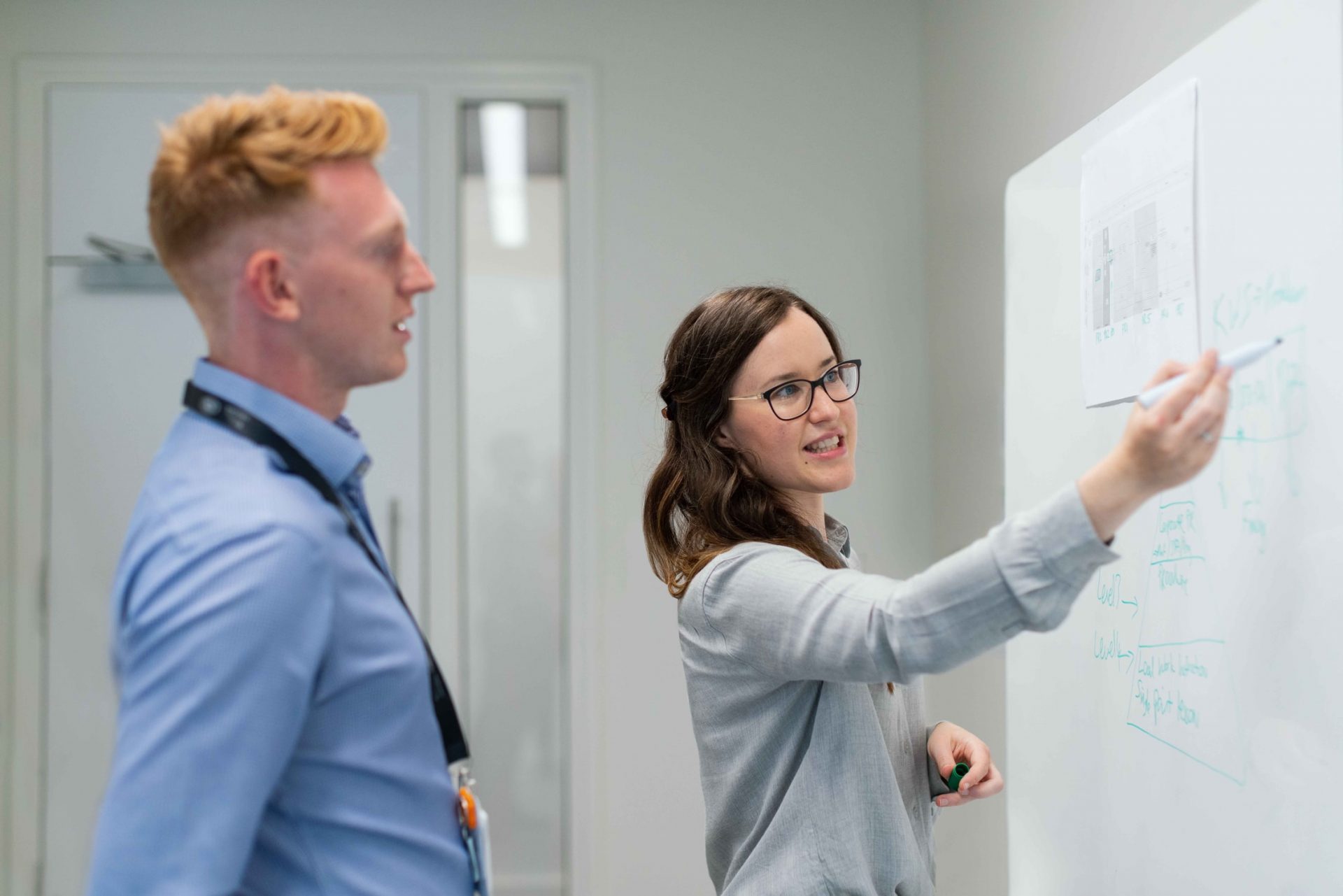
Hi, continuing with the post Dimensional Arrays explanation in this post I’m going to show you how to create a 2D Array ( two dimensional array) with Javascript code.
At the very least, we need to know the number of rows we want the array contain. With that information we can create a two-dimensional array with n numbers of rows and 1 column.
We can use this code approach:
let mainArray = [];
let rows = 3; // 3 rows
for (let i = 0; i < rows; i++) { // Creating each row
mainArray[i] = []; // Creating 1 column in each row
}
console.log('mainArray --->', mainArray);
Result: Matrix of 3 rows and 1 column – why? check Dimensional Arrays explanation

If we formatting this Matrix (mainArray) just for a better and understandable view this will be like this:
let mainArray = [ // 1 column
[ ], // 1 row
[ ], // 2 row
[ ] // 3 row
];
Now if we want to get a position value, the result will be ‘undefined‘:
console.log('mainArray position --->', mainArray[0][0]);
Result:

Ok, the problem with this approach is that each element of the array is set to ‘undefined’, so a better way to create a two-dimensional array is extends the Javascript Array OBJECT with a METHOD that sets THE NUMBER OF ROWS, NUMBER OF COLUMNS and sets the INITIAL VALUE passed in the method to every position in our matrix.
Like this:
// Extending the Array object
Array.matrix = (numRows, numColumns, initialValue) => { // Adding a method to the Array object
let matrix = []; // Is the array that will hold all the rows and columns = matrix or two-dimensional array
for(let i = 0; i < numRows; i++) { // Creating each row
let columns = []; // Because every column will be an Array type
// Creating the quantity of columns by each row
for (let j = 0; j < numColumns; j++) {
columns[j] = initialValue; // Saving initialValue in each position of the column
}
matrix[i] = columns; // Assiging all the columns in each row
}
return matrix; // When the loops done then the function returns the mainArray
};
let myMatrix = Array.matrix(3, 2, 'Cristina');
console.log('myMatrix -->', myMatrix);
Result: so this matrix is 3 columns ( 0 position + 1 position + 2 position = 3 positions) and 2 columns with initial value as ‘Cristina’

If we formatting this Matrix (mainArray) just for a better and understandable view this will be like this: 2 columns, 3 rows and all the positions with the initial value ‘Cristina’
myMatrix = [ // 1 column 2 column
['Cristina', 'Cristina'], // 1 row
['Cristina', 'Cristina'], // 2 row
['Cristina', 'Cristina'] // 3 row
];
So if we want to access to one position then we will have some data and this will not be returning ‘undefined‘ instead of this will return the data that we pass to the initialValue:

By Cristina Rojas.