Node Js course – 6 Importing npm Modules
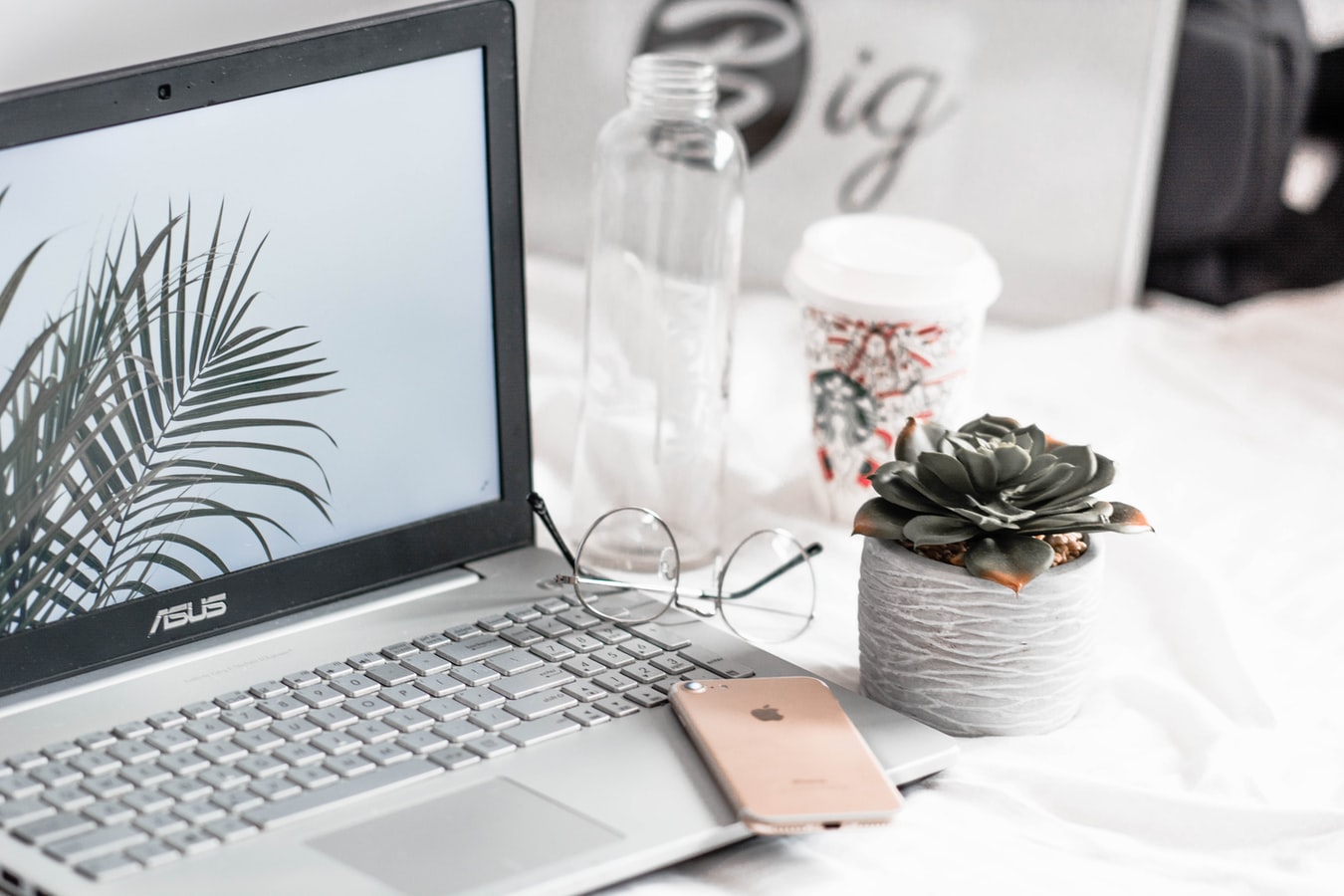
Hi, in this post we are going to learn how use the “module system” to load in “npm packages” this is going to allow us to take advantage of all npm modules from write inside in our Node applications.
When we install Node we also have a “npm” program install in our machine, this give us access to everything over a “npm js” and access to the npm command line tools.
Before we start using the npm packages we need to initialize npm in our project and then we need to install all of the modules that we actually want to use.
1.- Run this next command in the terminal to initialize npm in our project
npm init
This going to create a single configuration file that we can use to manage all of the dependencies.
The only thing that we need to do here is just press “Enter”.
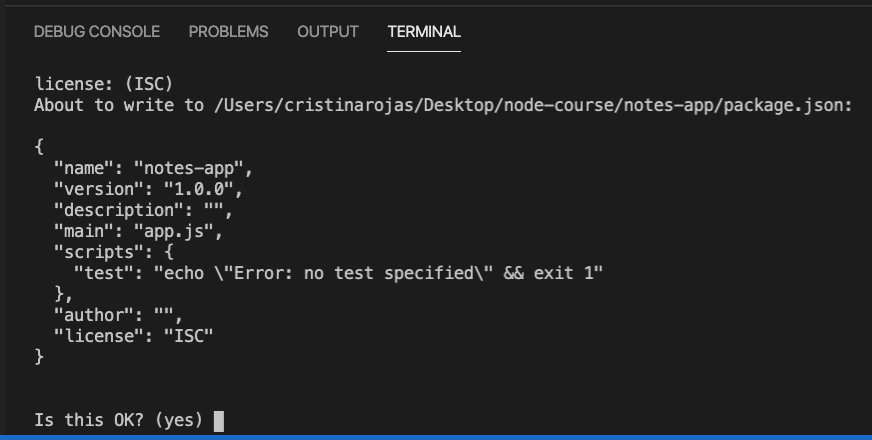
Note: I have a post that explain the steps.
And automatically we will see a “package.json” created inside our project.
Note: The extension of this file is “.json” that means JavaScript Object Notation.
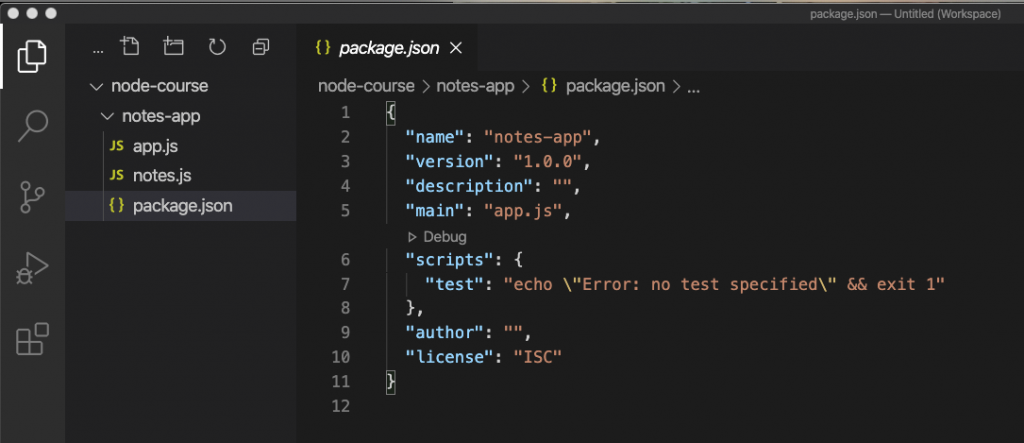
In this file we need to declare all the npm packages that the project need.
2.- Add the npm packages to the package.json
In this case the package will be:
To install this package run the next command in the terminal:
npm install validator@13.1.1
This command will go to the npm servers and will wrap all the code of that package and will be added into our application.
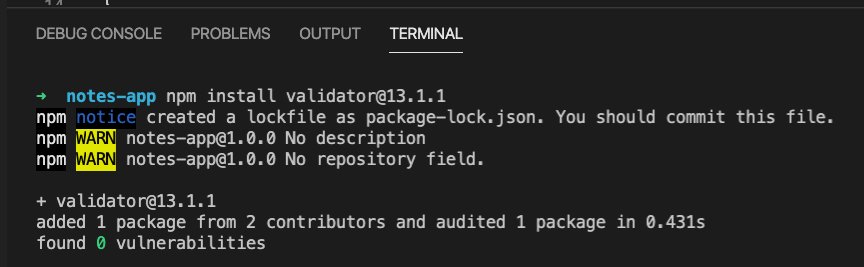
At this point 2 things happen in our project:
We have a file called “package-lock.json”: this file have extra information, making npm more faster and more secure and have information about all of our dependencies and where they are fetching from. This is not a file that we need to edit.
A folder called “node_modules”: this is the folder where have all the code of the dependencies (packages) that we installed, in this case will have the “validator” package
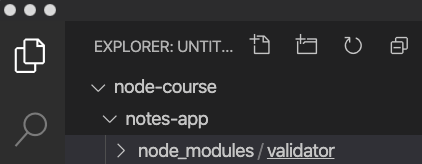
Note: we don’t need to change nothing here.
So, when we use “npm install” this maintains the “node_modules” folder.
Then if we see again our “package.json” we will see that the “validator” package is mentioned in the “dependencies” node.
"dependencies": {
"validator": "^13.1.1"
}
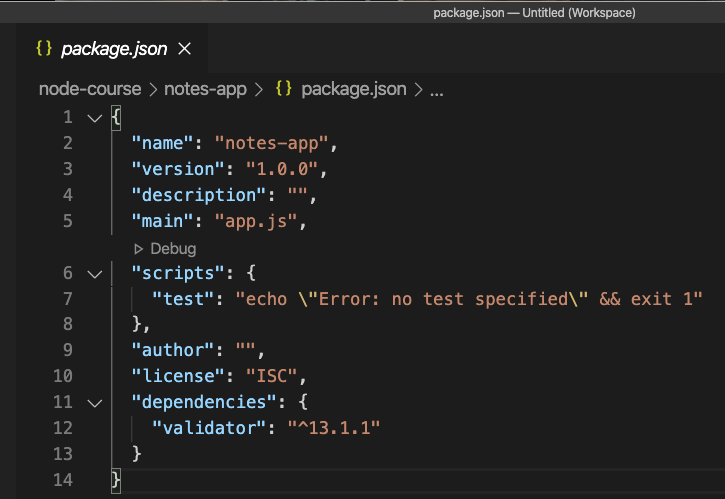
Finally we can import the “validator” package to the app.js and use .isEmail() method of that package to validate if a given email is valid or not.
app.js file will be like this:
// Dependencies
const validator = require('validator'); // the require will have all the return of the validator package.
// using isEmail method of validator package
// to check if is valid email
console.log(validator.isEmail('cristina@gmail.com'));
Result:

By Cristina Rojas.