The TypeScript type system
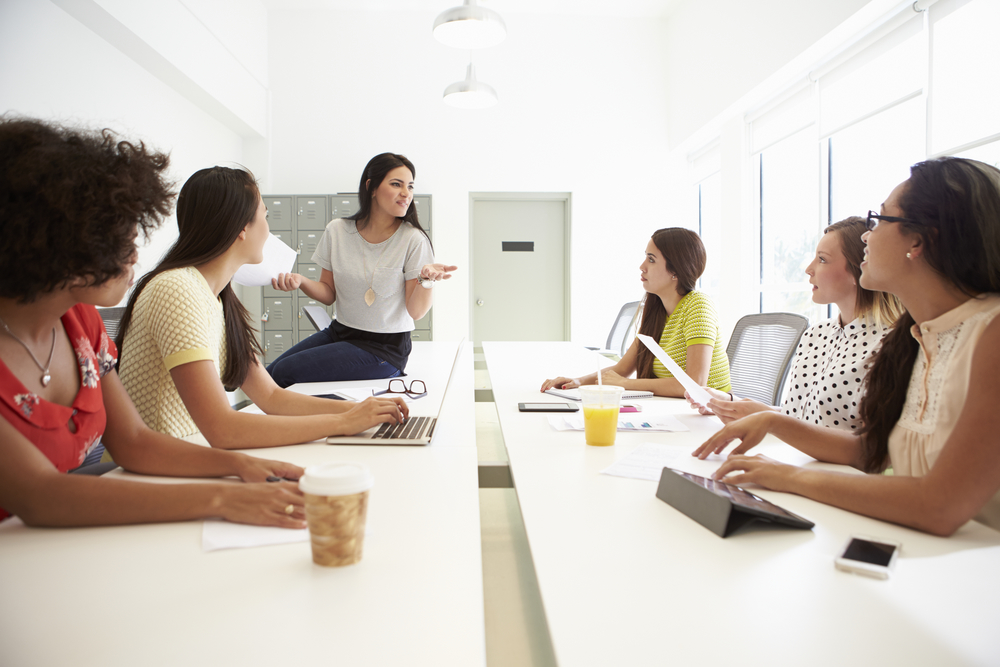
Hi, the Type system in TypeScript is a set of rules that a typechecker uses to assign types to your program.
To explicitly signal to TypeScript what your types are, use annotations.
Example:
let myNumber: number = 5; // myNumber is a number type!
let myName: string = "Cristina Rojas"; // myName is a string type!
let myArray: boolean[] = [false, true]; // myArray is an array of booleans
Differences between TypeScript and Javascript in the type system:
Types of bound?
Javascript is dynamically and TypeScript is Statically.
The types are automatically converted?
Javascript yes and TypeScript No(mostly)
When are types checked?
Javascript check types at runtime and TypeScript at compile time.
When are errors surfaced?
Javascript at runtime and TypeScript and compile time (mostly).
Ok, most places Javascript doesn’t care what types you give it, and instead Javascript tries to do the best to convert what you gave it to what it expects.
TypeScript statically analyze your code for errors (type errors) and show them to you before you run it.
For example, if we have a type errors then TypeScript will show us the errors in our editor (You can install a good TypeScript extension in your code editor, in this case I’m using VS code)
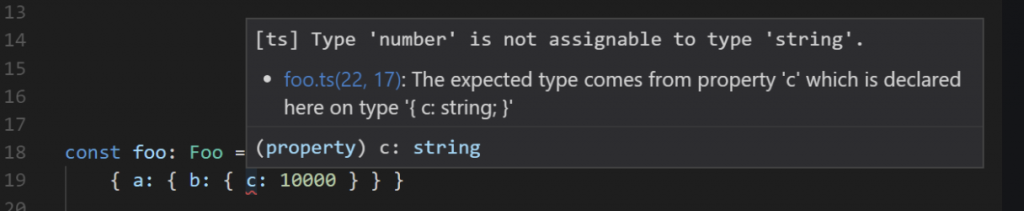
Nice! and one more thing that I wand to mention is that TypeScript can’t check for you at compile time thing like stack overflow, network connections, malformed user inputs, you will see that type of issues in runtime exceptions.
By Cristina Rojas.
Check my last post about TypeScript: